Write the program below to familiarize with the conventional syntax and formatting of Object-Oriented Programming in Python. 1. Let us create a class Dog with the script below. Save this script as dogs.py. Take note of the indentions, colons, and number of parenthesis used to avoid errors on our program. 1234567 2 68 def def roll(self): print (self.name.title() + rolled over!") def bark (self, times): print (self.name, 'says woof', times, ' times.') 2. Identify the user-defined attributes/parameters on our class Dog. 9 class Dog: definit_(self, name, breed, age): self.name = name self.breed self.age = age 10 11 12 breed sit(self): print (self.name.title() + is now sitting.") 3. Identify the user-defined methods or actions that can be acted out on our class Dog.
Write the program below to familiarize with the conventional syntax and formatting of Object-Oriented Programming in Python. 1. Let us create a class Dog with the script below. Save this script as dogs.py. Take note of the indentions, colons, and number of parenthesis used to avoid errors on our program. 1234567 2 68 def def roll(self): print (self.name.title() + rolled over!") def bark (self, times): print (self.name, 'says woof', times, ' times.') 2. Identify the user-defined attributes/parameters on our class Dog. 9 class Dog: definit_(self, name, breed, age): self.name = name self.breed self.age = age 10 11 12 breed sit(self): print (self.name.title() + is now sitting.") 3. Identify the user-defined methods or actions that can be acted out on our class Dog.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Programming Demo 3: Dog Class
Write the program below to familiarize with the conventional syntax and formatting of Object-Oriented
Programming in Python.
1. Let us create a class Dog with the script below. Save this script as dogs.py. Take note of the
indentions, colons, and number of parenthesis used to avoid errors on our program.
12345678
3
9
10
11
12
class Dog:
definit_(self, name, breed, age):
self.name = name
self.breed breed
=
self.age = age
def sit(self):
print (self.name.title() + is now sitting.")
123456
def roll(self):
"
print (self.name.title() + rolled over!")
def bark(self, times):
print (self.name, 'says woof', times, times.')
2. Identify the user-defined attributes/parameters on our class Dog.
"
3. Identify the user-defined methods or actions that can be acted out on our class Dog.
4. Create a new Python script and name it as sub_dogs.py. Make sure this script is saved on the same
folder with dogs.py. Write the codes below then run it.
from dogs import Dog
dog1= Dog("bantay", "aspin',4)
print (dog1.name)
print (dog1. breed)
print (dog1.age)

Transcribed Image Text:5. Determine the purpose of line 1 on our code. What will happen if we commented or delete the first
line before running the code.
6. Try replacing the values: "bantay" to "milo", 'aspin' to 'labrador', 4 to 13. What will be the outputs?
8
9
print('The name of my dog is + dog1.name.title() + ".")
print('She is an {0}. She is {1} years old.'
.format (dog1.breed.title(), str (dog1.age)))
10
11
7. Explain the outputs of line 8 to line 10.
dog1.sit()
dog1.roll()
dog1.bark()
12
13
14
15
8. Identify the outputs of line 12 to line 14. Try inserting an integer value inside the bark() function.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
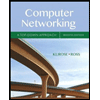
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
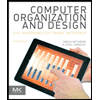
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
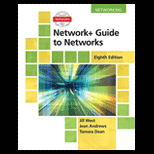
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
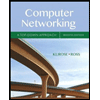
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
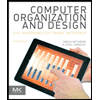
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
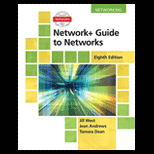
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
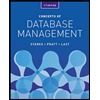
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
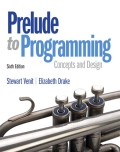
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
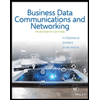
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY