Write the less-than operator and assume positive num. // ***********I cant seem to be getting the test to pass them all and i don't know why. Please help and explain******* Also i can only implement the '<' operator function********* Givent the header file: int.h (i cannot change this!!) class Int { public: Int(); bool operator<(const Int& rhs) const; private: string m_digits; }; int.cpp file: (i can change this please help me implement the function!) #include #include "int.h" using namespace std; bool Int::operator<(const Int& rhs) const { if (m_digits < rhs.m_digits) { return true; } } //this is the tester file I am given #include #include #include #include "int.h" using namespace std; int main() { cout << boolalpha; cout << R"(Int("999") < Int("1000"): )"; cout << (Int("999") < Int("1000")) << endl; cout << "Expected: true" << endl << endl; cout << R"(Int("999") < Int("998"): )"; cout << (Int("999") < Int("998")) << endl; cout << "Expected: false" << endl << endl; cout << R"(Int("998") < Int("999"): )"; cout << (Int("998") < Int("999")) << endl; cout << "Expected: true" << endl << endl; // operator < used for sorting vector v{{"9"}, {"7"}, {"5"}, {"12"}}; sort(v.begin(), v.end()); for (auto e : v) cout << e << " "; cout << "\nExpected: 5 7 9 12 " << endl; }
Write the less-than operator and assume positive num.
// ***********I cant seem to be getting the test to pass them all and i don't know why. Please help and explain******* Also i can only implement the '<' operator function*********
Givent the header file: int.h (i cannot change this!!)
class Int {
public: Int();
bool operator<(const Int& rhs) const;
private: string m_digits; };
int.cpp file: (i can change this please help me implement the function!)
#include <iostream>
#include "int.h"
using namespace std;
bool Int::operator<(const Int& rhs) const
{
if (m_digits < rhs.m_digits) { return true; }
}
//this is the tester file I am given
#include <iostream>
#include <vector>
#include <algorithm>
#include "int.h"
using namespace std;
int main()
{
cout << boolalpha;
cout << R"(Int("999") < Int("1000"): )";
cout << (Int("999") < Int("1000")) << endl;
cout << "Expected: true" << endl << endl;
cout << R"(Int("999") < Int("998"): )";
cout << (Int("999") < Int("998")) << endl;
cout << "Expected: false" << endl << endl;
cout << R"(Int("998") < Int("999"): )";
cout << (Int("998") < Int("999")) << endl;
cout << "Expected: true" << endl << endl;
// operator < used for sorting
vector<Int> v{{"9"}, {"7"}, {"5"}, {"12"}};
sort(v.begin(), v.end());
for (auto e : v) cout << e << " ";
cout << "\nExpected: 5 7 9 12 " << endl;
}


Step by step
Solved in 2 steps with 1 images

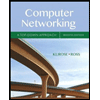
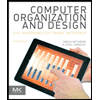
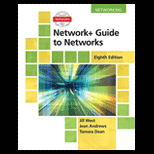
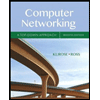
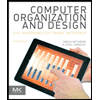
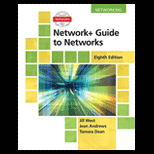
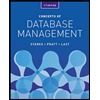
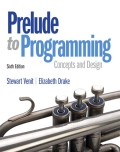
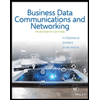