write the following for loop as a while loop
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
write the following for loop as a while loop

Transcribed Image Text:The following is a transcription of a C++ function, which simulates part of a shooting mechanism within a game. The function is defined for a Player class. The primary purpose of this function is to determine if a shot successfully hits a target, specifically targeting robots in an arena.
```cpp
bool Player::shoot(int dir)
{
m_age++;
if (rand() % 3 == 0) // miss with 1/3 probability
{
return false;
}
else if (dir == UP)
{
for (int i = 0; i < MAXSHOTLEN + 1; i++)
{
if (m_arena->nRobotsAt(m_row - i, m_col) > 0 && m_row - i > 0)
{
m_arena->damageRobotAt(m_row - i, m_col);
return true;
}
}
}
}
```
### Explanation:
1. **Function Signature**:
- `bool Player::shoot(int dir)`
- This defines a member function `shoot` of the `Player` class, which takes an integer `dir` representing the direction of the shot and returns a boolean value indicating success or failure.
2. **Age Increment**:
- `m_age++`: Increases the age of the player with every shot attempt.
3. **Probability of Missing**:
- `if (rand() % 3 == 0)`: Simulates a 1/3 probability of the shot missing, as `rand() % 3` will equal zero approximately one-third of the time.
- If the shot is a miss, the function returns `false`.
4. **Directional Shooting**:
- `else if (dir == UP)`: Handles the case when the shot direction is upwards.
- A `for` loop iterates up to `MAXSHOTLEN + 1`, representing the maximum shot length.
5. **Checking for Robots**:
- Inside the loop, `if (m_arena->nRobotsAt(m_row - i, m_col) > 0 && m_row - i > 0)`: Checks if any robots are at the position `m_row - i, m_col` and ensures the row index remains valid.
- If a robot is present, `m_arena->damageRobotAt(m_row - i, m_col);` damages
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
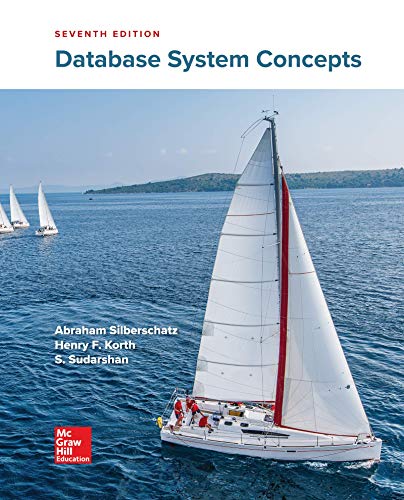
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
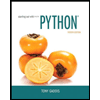
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
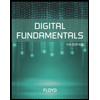
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
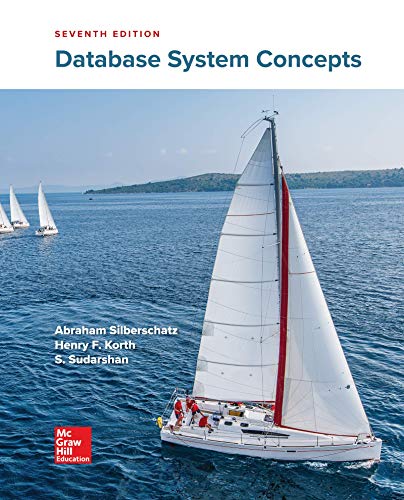
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
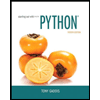
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
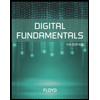
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
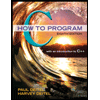
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
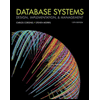
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
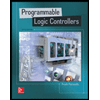
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education