Write segments out to the 7-segment display spi_write(segments); }
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter8: I/o Streams And Data Files
Section8.1: I/o File Stream Objects And Functions
Problem 6E
Question
* We have declared some functions in "timer.c" and corresponding function
* prototypes in "timer.h":
* void timer_init(void); // Initialise TCB0 to produce interrupts every 1ms
*
* Your task for Ex 9.3 is to complete the ISR declared in "timer.c" to display
* simultaneously the first digit of your student number on the LHS of the 7-segment
* display and the second digit of your student number on the RHS of this display (only
* after timer_init() is called).
*
* TIP: Every time a new interrupt occurs you should swap which digit you are displaying.
*
* TIP: You will want to declare a global or static variable so you know which digit to
* display on each iteration.
*
* TIP: You might like to refer to the following truth table for 7-segment digit mapping.
* (remember to check which output Q0..Q6 maps to which segment on the QUTy)
*
* ABCDEFG
* 0: 0000001
* 1: 1001111
* 2: 0010010
* 3: 0000110
* 4: 1001100
* 5: 0100100
* 6: 0100000
* 7: 0001111
* 8: 0000000
* 9: 0000100
#include <avr/io.h>
#include "spi.h"
static uint8_t current_digit = 0;
ISR(TCB0_INT_vect) {
// Determine which digit to display
uint8_t digit = current_digit ? (11233184 % 100) / 10 : 11233184 % 10;
current_digit = !current_digit;
// Map digit to 7-segment display
uint8_t segments = 0;
switch (digit) {
case 0:
segments = 0b0000001;
break;
case 1:
segments = 0b1001111;
break;
case 2:
segments = 0b0010010;
break;
case 3:
segments = 0b0000110;
break;
case 4:
segments = 0b1001100;
break;
case 5:
segments = 0b0100100;
break;
case 6:
segments = 0b0100000;
break;
case 7:
segments = 0b0001111;
break;
case 8:
segments = 0b0000000;
break;
case 9:
segments = 0b0000100;
break;
}
// Write segments out to the 7-segment display
spi_write(segments);
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
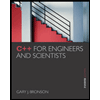
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
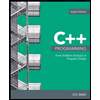
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
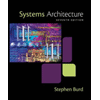
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
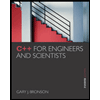
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
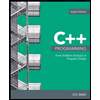
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
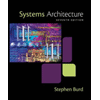
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
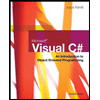
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,