Write in C language Description You have 2 tables, courses and scores. courses consists 3 columns, sid, student_id, and course_id. scores consists 2 columns,sid and score. Please find that how many students failed on at least one course. Input First line consists 2 integers m and n, shows number of rows in courses and scores. Followed m lines are records of courses. student_id are 8-digits numbers begin with non-zero digit. sid and scores are positive integers smaller than 1000. Followed n lines are records of scores. sid are positive integers smaller than 1000. score are integers in range [0, 100]. Output
Write in C language
Description
You have 2 tables, courses and scores. courses consists 3 columns, sid, student_id, and course_id. scores consists 2 columns,sid and score. Please find that how many students failed on at least one course.
Input
First line consists 2 integers m and n, shows number of rows in courses and scores.
Followed m lines are records of courses. student_id are 8-digits numbers begin with non-zero digit. sid and scores are positive integers smaller than 1000.
Followed n lines are records of scores. sid are positive integers smaller than 1000. score are integers in range [0, 100].
Output
Student will be flunked when his/her average score in the course less than 60. Output is 2 integers shows number of student and student failed on at lease one course.
Sample Input 1
2 4Sample Output 1
2 1Expert Answer (Sample Input and Output need to be the same as the input and output nothing else)
There are 2 tables, courses and scores. The courses consists of 3 columns, sid, student_id, and course_id whereas scores consists 2 columns, sid and score.
The ask is to find out the total number of students and the number of students who have failed in atleast one course.
The scores table contains multiple scores of a student for a course. So, we would need to find out the average score obtained in a given course for a student.
So, we would need to start with defining 2-D arrays to store the courses and scores details.
#include<stdio.h>
int main(){
/* 2D array declaration*/
int m=0;
int n=0;
printf("Enter value for m:");
scanf("%d",&m);
printf("Enter value for m:");
scanf("%d",&n);
int course[m][3];
/*Counter variables for the loop*/
int i, j;
for(i=0; i<m; i++) {
for(j=0;j<3;j++) {
printf("Enter value for course[%d][%d]:", i, j);
scanf("%d", &course[i][j]);
}
}
int score[n][2];
/*Counter variables for the loop*/
for(i=0; i<n; i++) {
for(j=0;j<3;j++) {
printf("Enter value for score[%d][%d]:", i, j);
scanf("%d", &score[i][j]);
}
}
int prevSid=0, prevScore=0, noOfOccurence=0, noOfFailedStudents=0;
for(i=0;i<n; i++) {
sid = score[i][0];
if(prevSid==sid) {
totalScore=prevScore+score[i][1];
prevSid=sid; noOfOccurence++;
} else {
if(totalScore/noOfOccurence<60) {
noOfFailedStudents++;
}
prevSid=0; prevScore=0;
}
}
printf("Output: ");
printf("%d", m);
printf("%d", noOfFailedStudents);
return 0;
}

Step by step
Solved in 2 steps

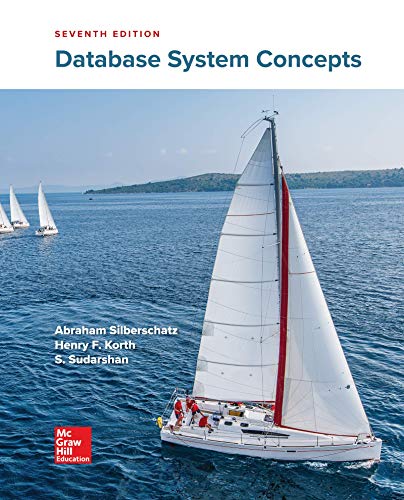
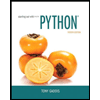
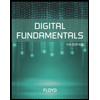
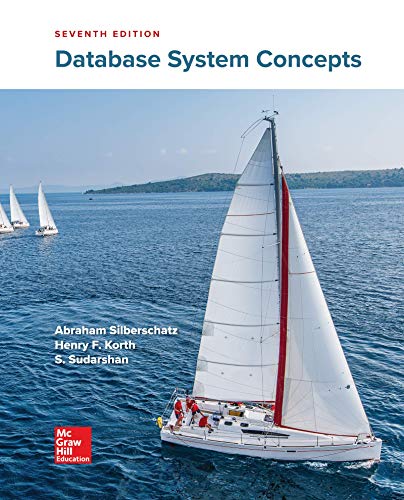
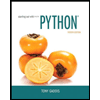
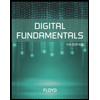
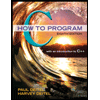
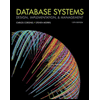
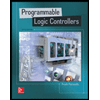