Write C++ program to do arithmetic operations (+), (-), (/) and (*) usir function and switch case?


I have implemented the given requirements as per the specification in the C++ language. Comments are mentioned for better understanding of the code.The code is as follows:
#include <iostream>
#include <stdio.h>
#include <stdlib.h>
using namespace std;
//Function to calculate sum
float sum(float a, float b){
float sum = a + b;
return sum;
}
//Function to calculate difference
float difference(float a, float b){
float diff = a - b;
return diff;
}
//Function to calculate product
float product(float a, float b){
float p = a * b;
return p;
}
//Function to calculate quotient
float quotient(float a, float b){
float quo = a / b;
return quo;
}
int main()
{
char choice;
float a, b, result;
//Menu display
cout << "\n1. Enter (+) for sum\n2. Enter (-) for difference\n3. Enter (*) for product\n4. Enter (/) for quotient\n5. Exit\nEnter your choice : ";
cin >> choice;
//Switch case
switch(choice){
case '+':
//Taking the values input from the use
cout << "Enter first value : ";
cin >> a;
cout << "Enter second value : ";
cin >> b;
//Calling the respective function with the input values as parameters
result = sum(a, b);
cout << "\nResult : " << result;
break;
case '-':
cout << "Enter first value : ";
cin >> a;
cout << "Enter second value : ";
cin >> b;
result = difference(a, b);
cout << "\nResult : " << result;
break;
case '*':
cout << "Enter first value : ";
cin >> a;
cout << "Enter second value : ";
cin >> b;
result = product(a, b);
cout << "\nResult : " << result;
break;
case '/':
cout << "Enter first value : ";
cin >> a;
cout << "Enter second value : ";
cin >> b;
result = quotient(a, b);
cout << "\nResult : " << result;
break;
case 5:
exit(1);
}
return 0;
}
Step by step
Solved in 2 steps with 1 images

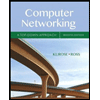
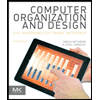
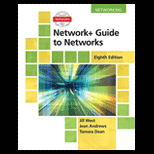
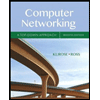
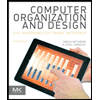
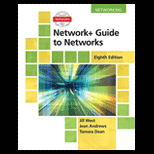
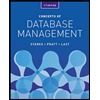
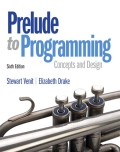
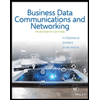