Write an MPI program 'Estimate-Pi.c' from the scratch to estimate the value of Pi (π) using a Monte Carlo method. Your program should perform necessary error checking for inputs.
Write an MPI program 'Estimate-Pi.c' from the scratch to estimate the value of Pi (π) using a Monte Carlo method. Your program should perform necessary error checking for inputs.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Write an MPI

Transcribed Image Text:This is called a “Monte Carlo" method, since it uses randomness (the dart tosses).
Specifically, the MPI program can be implemented in the following way.
(1) Process 0 should read in the total number of tosses and broadcast it to the other processes.
(2) Use MPI_Reduce to find the global sum of the local variable number_in_circle, and have
process 0 print the result.
(3) You may want to use long long ints for the number of hits in the cirele and the number
of tosses, since both may have to be very large to get a reasonable estimate of n.
1.

Transcribed Image Text:Write an MPI program that uses a Monte Carlo method to estimate a. Suppose we toss darts
randomly at a square dartboard, whose bullseye is at the origin, and whose sides are 2 feet in
length. Suppose also that there's a circle inscribed in the square dartboard. The radius of the
circle is 1 foot, and its area is a square feet. If the points that are hit by the darts are
uniformly distributed (and we always hit the square), then the number of darts that hit inside
the circle should approximately satisfy the equation
number in circle
total number of tosses
4
since the ratio of the area of the circle to the area of the square is a/4.
We can use this formula to estimate the value of a with a random number generator:
number_in_circle = 0;
for (toss = 0; toss <number_of_tosses; toss++) {
x= random double between -1 and 1;
y= random double between -1 and 1;
distance_squared =x*x+ y*y;
if (distance_squared <= 1) number_in_cirele++;
}
pi_estimate = 4*number_in_circle/((double) number_of_tosses);
This is called a “Monte Carlo" method, since it uses randomness (the dart tosses).
Specifically, the MPI program can be implemented in the following way.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
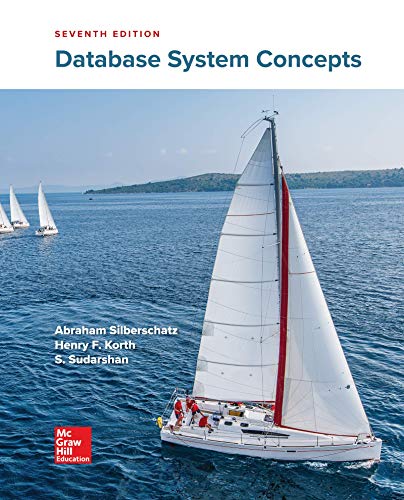
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
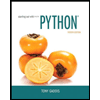
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
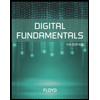
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
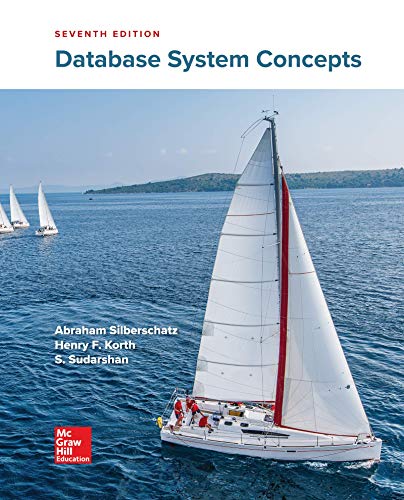
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
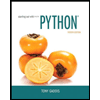
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
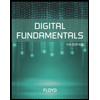
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
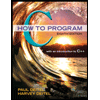
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
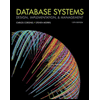
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
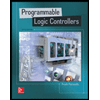
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education