Write a substitution code for an alphabetic string that converts both lower and upper case letters. As with the previous question declare a permutation of the 26 alphabetic characters i.e. char perm[] = “qjczieaungsdfxmphybklortvw” Now, given a message string e.g.
Write a substitution code for an alphabetic string that converts both lower and upper case letters.
As with the previous question declare a permutation of the 26 alphabetic characters i.e.
char perm[] = “qjczieaungsdfxmphybklortvw”
Now, given a message string e.g.
char msg[] = “secret message to encode”;
Replace all occurrences of ‘a’ with ‘q’ (since perm[0]=’q’)
Replace all occurrences of ‘b’ with ‘j’ (since perm[1]=’j’)
…
Replace all occurrences of ‘z’ with ‘w’ (since perm[25]=’w’)
So that, the message is modified to
“bicyik fibbqai km ixcmzi”
In this case your solution also handle uppercase characters, so that if
“Secret Message To Encode”;
the message is modified to
“Bicyik Fibbqai Km Ixcmzi”
For example:
Input | Result |
---|---|
secret message to encode | bicyik fibbqai km ixcmzi |
Secret Message to Encode | Bicyik Fibbqai km Ixcmzi |
Code i was given:
#include <stdio.h>
#define MAX_STR_LEN 256
int main(void)
{
/* keep the perm[] array fixed as it is here, though
your program should work for any permutation array */
char perm[] = "qjczieaungsdfxmphybklortvw";
char msg[MAX_STR_LEN+1];
/* read the message from the terminal using fgets. The variable msg will contain the message. */
fgets(msg, MAX_STR_LEN, stdin);
/* write your answer here */
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

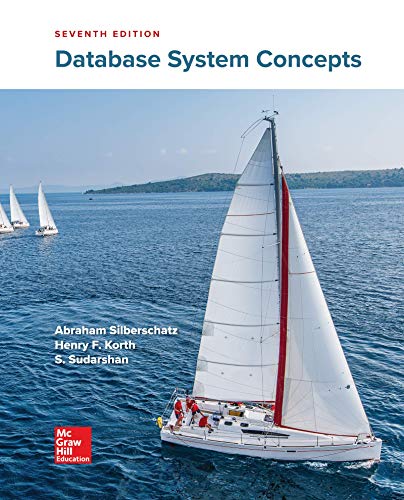
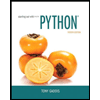
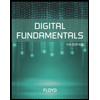
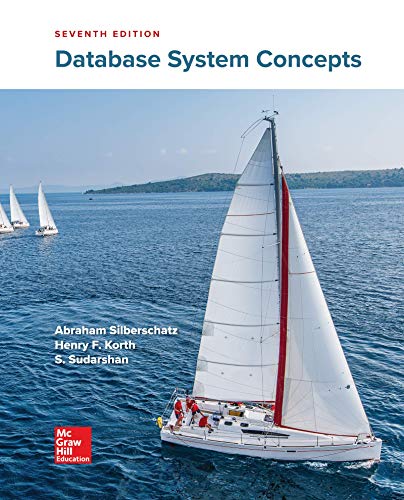
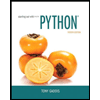
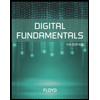
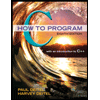
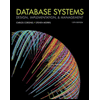
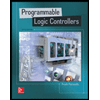