Write a static method called removeMatches that removes extra copies of a value that are adjacent to it in an ArrayList of Integer objects. For example, if a variable called list contains the following: [1, 3, 3, 3, 3, 15, 2, 2, 1, 19, 3, 42, 42, 42, 7, 42, 1] and we make the following call: removeMatches (list); Then list should store the following values after the call: [1, 3, 15, 2, 1, 19, 3, 42, 7, 42, 1]
Write a static method called removeMatches that removes extra copies of a value that are adjacent to it in an ArrayList of Integer objects. For example, if a variable called list contains the following: [1, 3, 3, 3, 3, 15, 2, 2, 1, 19, 3, 42, 42, 42, 7, 42, 1] and we make the following call: removeMatches (list); Then list should store the following values after the call: [1, 3, 15, 2, 1, 19, 3, 42, 7, 42, 1]
Chapter9: Advanced Array Concepts
Section: Chapter Questions
Problem 18RQ
Related questions
Question
How do you do this? JAVA
![Next
Description
Write a static method called removeMatches that removes
extra copies of a value that are adjacent to it in an ArrayList
of Integer objects. For example, if a variable called list
contains the following:
[1, 3, 3, 3, 3, 15, 2, 2, 1, 19, 3, 42, 42, 42, 7, 42, 1]
and we make the following call:
remove Matches (list);
Then list should store the following values after the call:
[1, 3, 15, 2, 1, 19, 3, 42, 7, 42, 1]
Notice that the four bccurrences of 3 that appear next to each
other have been replaced with a single occurrence of 3, the
two occurrences of 2 that appear next to each other have been
replaced with a single 2, and the three occurrences of 42 that
appear next to each other have been replaced with a single 42.
Notice, however, that the list still contains three occurrences of
1, two occurrences of 3, and two occurrences of 42. That is
because those duplicate values do not appear right next to
each other in the list.
You may not construct any extra data structures to solve this
problem. You must solve it by manipulating the ArrayList
you are passed as a parameter.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdb5f8f02-6709-43da-9aaa-e09884bfc964%2F1521886f-63d5-4ca0-94d5-1197abc27a45%2Fw4owyoa_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Next
Description
Write a static method called removeMatches that removes
extra copies of a value that are adjacent to it in an ArrayList
of Integer objects. For example, if a variable called list
contains the following:
[1, 3, 3, 3, 3, 15, 2, 2, 1, 19, 3, 42, 42, 42, 7, 42, 1]
and we make the following call:
remove Matches (list);
Then list should store the following values after the call:
[1, 3, 15, 2, 1, 19, 3, 42, 7, 42, 1]
Notice that the four bccurrences of 3 that appear next to each
other have been replaced with a single occurrence of 3, the
two occurrences of 2 that appear next to each other have been
replaced with a single 2, and the three occurrences of 42 that
appear next to each other have been replaced with a single 42.
Notice, however, that the list still contains three occurrences of
1, two occurrences of 3, and two occurrences of 42. That is
because those duplicate values do not appear right next to
each other in the list.
You may not construct any extra data structures to solve this
problem. You must solve it by manipulating the ArrayList
you are passed as a parameter.
![+
RemoveMatches.java
1 import java.util.*;
2
3 public class RemoveMatches {
4
5
6
public static void main(String[] args) {
Integer [] test1 = {1, 3, 3, 3, 3, 15, 2, 2, 1, 19, 3, 42, 42, 42, 7, 42, 1};
ArrayList<Integer> list = new ArrayList<Integer> (Arrays.asList (test1));
System.out.println("Before:
" + list);
removeMatches (list);
System.out.println("After : " + list);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fdb5f8f02-6709-43da-9aaa-e09884bfc964%2F1521886f-63d5-4ca0-94d5-1197abc27a45%2Fen7hfgj_processed.jpeg&w=3840&q=75)
Transcribed Image Text:+
RemoveMatches.java
1 import java.util.*;
2
3 public class RemoveMatches {
4
5
6
public static void main(String[] args) {
Integer [] test1 = {1, 3, 3, 3, 3, 15, 2, 2, 1, 19, 3, 42, 42, 42, 7, 42, 1};
ArrayList<Integer> list = new ArrayList<Integer> (Arrays.asList (test1));
System.out.println("Before:
" + list);
removeMatches (list);
System.out.println("After : " + list);
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
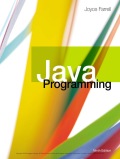
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
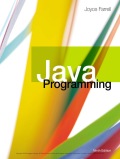
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT