Write a recursive C++ program that will output all the subsets of a set of n elements (without repeating any subsets).
Write a recursive C++ program that will output all the subsets of a set of n elements (without repeating any subsets).

Code:
#include<iostream>//header files
#include<vector>
#include<cmath>
#include<algorithm>
#include<climits>
#include<string>
#include<queue>
#include<stack>
#include<set>
using namespace std;
set<string> st;
void fun(int n, int const m, vector<string> &vec, string s){//function definition
if(n>=m){//if the size of the elements greater than m
s = s.substr(0,s.length()-1);//then s is substring of length - 1
if(st.find(s) == st.end()){//if you find s in substring then insert s
st.insert(s);
}
return;
}
fun(n+1,m,vec,s);//calling the function
fun(n+1,m,vec,s + vec[n] + " ");//calling the function
}
vector<string> vec;//defining vector
int main()
{
int t=0;
vec.clear(); st.clear();//clearing vector
int n=0;
cout<<"Enter size of the elements :";
cin >> n;
string s="";
cout<<"Enter elements of the set :";
for(int i=0;i<n;i++){
cin >> s;
vec.push_back(s);
}
cout<<"\nTotal subsets are:";
sort(vec.begin(), vec.end());//sorting vector
fun(0,n,vec,"");//calling the function
while(!st.empty()){//condition checing whether st is empty
cout << '(' << *st.begin() << ')';
st.erase(st.begin());
}
cout << endl;
return 0;
}
Code Screenshot:
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

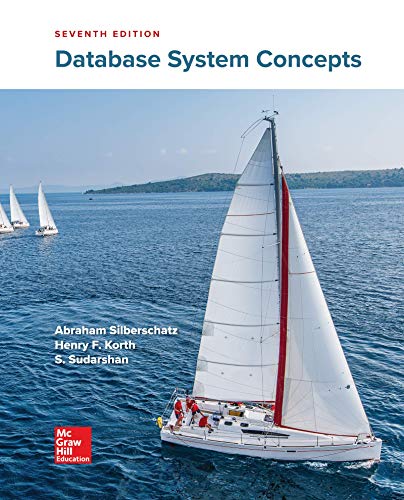
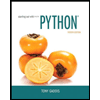
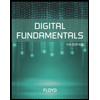
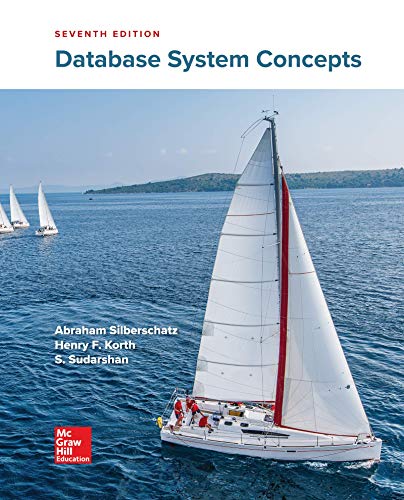
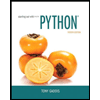
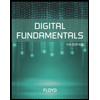
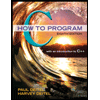
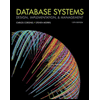
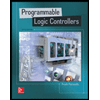