Write a Python program to take a string from user and remove all the duplicate characters from it where the order must be same.
Write a Python program to take a string from user and remove all the duplicate characters from it where the order must be same.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![### Removing Duplicate Characters from a String using Python
#### Task Description:
Write a Python program to take a string from the user and remove all the duplicate characters from it, while ensuring the order of characters remains the same.
#### Example:
Input: `"programming"`
Output: `"progamin"`
#### Explanation:
In the provided example, the string `"programming"` has the following duplicate characters: `r`, `g`, and `m`. The program will remove the second occurrence of each duplicate character while preserving the order of their first appearances.
#### Sample Solution Code:
```python
def remove_duplicates(input_string):
seen = set()
result = []
for char in input_string:
if char not in seen:
seen.add(char)
result.append(char)
return ''.join(result)
# Taking input from user
user_input = input("Enter a string: ")
print("String after removing duplicates:", remove_duplicates(user_input))
```
#### How it Works:
1. **Initialization**:
- `seen` is a set that will keep track of characters that have already appeared in the string.
- `result` is a list that will store characters in the order they first appear.
2. **Iteration Over Input String**:
- The program iterates through each character of the input string.
- If the character has not been seen before (`if char not in seen:`), it is added to the `seen` set and also appended to the `result` list.
3. **Final Output**:
- After the iteration is complete, the `result` list will contain all characters of the original string but without duplicates while preserving the original order.
- The `result` list is then converted into a string using `join()` method and returned as the final result.
#### Usage:
1. **Run the Program**: Execute the Python script in a Python environment.
2. **Input the String**: When prompted, enter the string from which you want to remove duplicate characters.
3. **View Output**: The processed string with duplicates removed will be printed on the console.
#### Additional Information:
- This algorithm has a time complexity of O(n), where n is the length of the input string, making it efficient for moderately sized inputs.
- The space complexity is also O(n), due to the extra storage required for the `seen` set and `result` list.
This method ensures the order is preserved and duplicates are](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc85aa270-dbe7-4d43-853e-3272fa6f5f37%2F92bc30db-4238-40f2-a166-4fc74dc4cb57%2Fhkunytw_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Removing Duplicate Characters from a String using Python
#### Task Description:
Write a Python program to take a string from the user and remove all the duplicate characters from it, while ensuring the order of characters remains the same.
#### Example:
Input: `"programming"`
Output: `"progamin"`
#### Explanation:
In the provided example, the string `"programming"` has the following duplicate characters: `r`, `g`, and `m`. The program will remove the second occurrence of each duplicate character while preserving the order of their first appearances.
#### Sample Solution Code:
```python
def remove_duplicates(input_string):
seen = set()
result = []
for char in input_string:
if char not in seen:
seen.add(char)
result.append(char)
return ''.join(result)
# Taking input from user
user_input = input("Enter a string: ")
print("String after removing duplicates:", remove_duplicates(user_input))
```
#### How it Works:
1. **Initialization**:
- `seen` is a set that will keep track of characters that have already appeared in the string.
- `result` is a list that will store characters in the order they first appear.
2. **Iteration Over Input String**:
- The program iterates through each character of the input string.
- If the character has not been seen before (`if char not in seen:`), it is added to the `seen` set and also appended to the `result` list.
3. **Final Output**:
- After the iteration is complete, the `result` list will contain all characters of the original string but without duplicates while preserving the original order.
- The `result` list is then converted into a string using `join()` method and returned as the final result.
#### Usage:
1. **Run the Program**: Execute the Python script in a Python environment.
2. **Input the String**: When prompted, enter the string from which you want to remove duplicate characters.
3. **View Output**: The processed string with duplicates removed will be printed on the console.
#### Additional Information:
- This algorithm has a time complexity of O(n), where n is the length of the input string, making it efficient for moderately sized inputs.
- The space complexity is also O(n), due to the extra storage required for the `seen` set and `result` list.
This method ensures the order is preserved and duplicates are
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
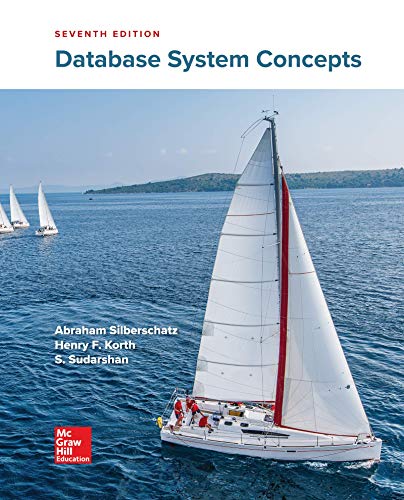
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
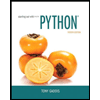
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
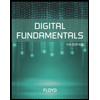
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
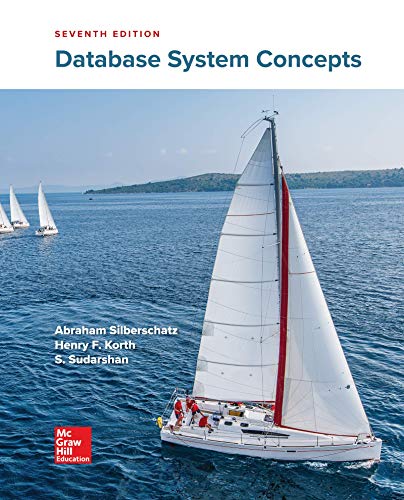
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
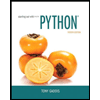
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
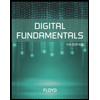
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
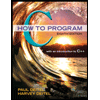
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
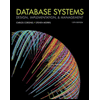
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
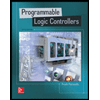
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education