Write a python program that takes the CGPA and no of credits completed by a student and prints whether the student is eligible for a waiver and of what percentage. To be eligible for a waiver, a student must have completed at least 30 credits and earned a CGPA greater or equal to 3.8. If not, please print "The student not eligible for a waiver". CGPA Waiver percentage 25 percent 50 percent 75 percent 100 percent 3.80 3.89 3.90-3.94 3.95- 3.99 100
Write a python program that takes the CGPA and no of credits completed by a student and prints whether the student is eligible for a waiver and of what percentage. To be eligible for a waiver, a student must have completed at least 30 credits and earned a CGPA greater or equal to 3.8. If not, please print "The student not eligible for a waiver". CGPA Waiver percentage 25 percent 50 percent 75 percent 100 percent 3.80 3.89 3.90-3.94 3.95- 3.99 100
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:### Eligibility for Waiver Based on CGPA and Completed Credits
This tutorial outlines how to write a Python program that determines if a student is eligible for a tuition waiver based on their Cumulative Grade Point Average (CGPA) and the number of completed credits. The program will also identify the percentage of the waiver the student qualifies for, if any.
#### Eligibility Criteria
To be eligible for a waiver, the student must meet the following requirements:
1. Must have completed at least 30 credits.
2. Must have earned a CGPA equal to or greater than 3.8.
If the student does not meet either of these conditions, the program should print:
```
"The student is not eligible for a waiver."
```
#### Waiver Percentage Based on CGPA
The following table displays the waiver percentages corresponding to different CGPA ranges:
| CGPA | Waiver Percentage |
|------------|--------------------|
| 3.80 - 3.89| 25 percent |
| 3.90 - 3.94| 50 percent |
| 3.95 - 3.99| 75 percent |
| 4.00 | 100 percent |
This information can be used to modify the program logic as required to calculate the appropriate waiver percentage once a student is deemed eligible.
### Example of Python Program
Here is an example of a Python program that implements the above logic:
```python
def waiver_eligibility(cgpa, credits):
if credits < 30:
return "The student is not eligible for a waiver."
if cgpa >= 3.80 and cgpa < 3.90:
return "The student is eligible for a 25 percent waiver."
elif cgpa >= 3.90 and cgpa < 3.95:
return "The student is eligible for a 50 percent waiver."
elif cgpa >= 3.95 and cgpa < 4.00:
return "The student is eligible for a 75 percent waiver."
elif cgpa == 4.00:
return "The student is eligible for a 100 percent waiver."
else:
return "The student is not eligible for a waiver."
# Example usage:
cgpa = float(input("Enter the CGPA: "))
credits = int(input("Enter the number of completed credits: "))
print(waiver_eligibility(cgpa, credits
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
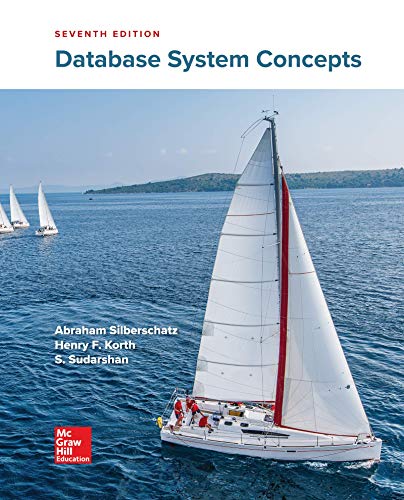
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
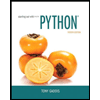
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
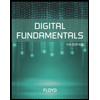
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
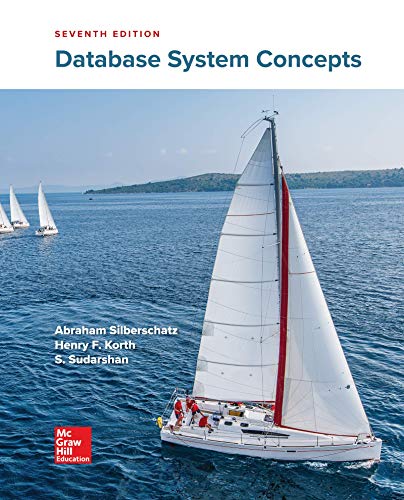
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
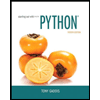
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
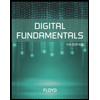
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
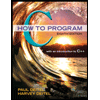
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
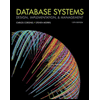
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
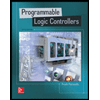
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education