Write a Python function def isSubArray(A,B) which takes two arrays and returns True if the first array is a (contiguous) subarray of the second array, otherwise it returns False. You may solve this problem using recursion or iteration or a mixture of recursion and iteration.
Write a Python function
def isSubArray(A,B)
which takes two arrays and returns True if the first array is a (contiguous) subarray of the second array, otherwise it returns False. You may solve this problem using recursion or iteration or a mixture of recursion and iteration.
For an array to be a subarray of another, it must occur entirely within the other one without other elements in between. For example:
- [31,7,25] is a subarray of [10,20,26,31,7,25,40,9]
- [26,31,25,40] is not a subarray of [10,20,26,31,7,25,40,9]
A good way of solving this problem is to make use of an auxiliary function that takes two arrays and returns True if the contents of the first array occur at the front of the second array, otherwise it returns False. Then, A is a subarray of B if it occurs at the front of B, or at the front of B[1:], or at the front of B[2:], etc. Note you should not use A == B for arrays.
![Write a Python function
def isSubArray (A, B)
which takes two arrays and returns True if the first array is a (contiguous) subarray of the second array, otherwise it returns False . You may solve this
problem using recursion or iteration or a mixture of recursion and iteration.
For an array to be a subarray of another, it must occur entirely within the other one without other elements in between. For example:
• [31,7, 25] is a subarray of [10,20, 26,31,7,25,40,9]
[26,31, 25,40] is not a subarray of [10,20,26,31,7,25,40,9]
Hint: A good way of solving this problem is to make use of an auxiliary function that takes two arrays and returns True if the contents of the first array occur at
the front of the second array, otherwise it returns False. Then, A is a subarray of B if it occurs at the front of B, or at the front of B[1:], or at the front of B[2:], etc.
Note you should not use A == B for arrays.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff756170d-2d1d-4a13-8a12-5e3152a86d82%2F1d0c6ed9-75d4-4dbb-b3cb-8315906a7edb%2Fkrunifd_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps with 2 images

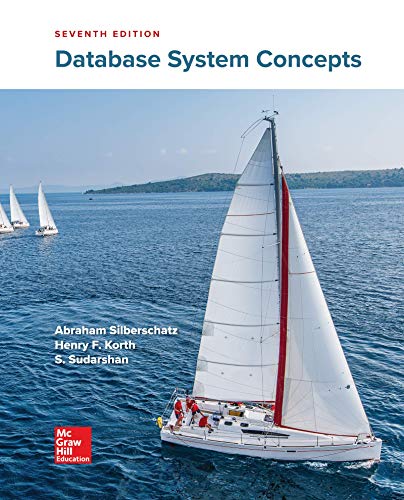
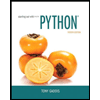
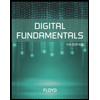
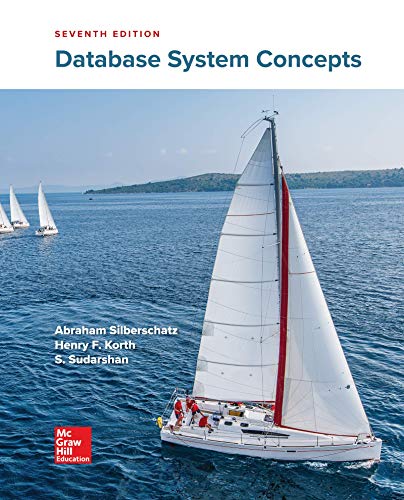
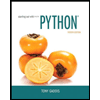
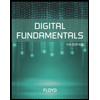
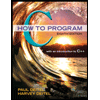
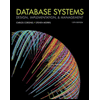
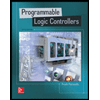