write a pseudocode for this programme in c++
write a pseudocode for this programme in c++
#include<iostream>
#include<string.h>
using namespace std;
struct staff_info
{
char name[40];
int noIC;
int age;
int category;
int scheme;
};
int main()
{
struct staff_info staff[10];
for(int i=0;i<10;i++)
{
cout<<"Enter Name: ";
cin>>staff[i].name;
cout<<"Enter IC Number: ";
cin>>staff[i].noIC;
cout<<"Enter Age: ";
cin>>staff[i].age;
cout<<"Enter Category(Press 1 for Academic / 2 for Non-Academic): ";
cin>>staff[i].category;
cout<<"Enter Scheme (Press 1 for Pension on 56 years / 2 for Pension on 60 years: ";
cin>>staff[i].scheme;
}
cout<<endl;
cout<<"Staff's Information"<<endl;
cout<<"Name\t\tNoIC\t\tAge\t\tCategory\t\tScheme"<<endl;
for(int i=0;i<10;i++)
{
cout<<staff[i].name<<"\t";
cout<<staff[i].noIC<<"\t";
cout<<staff[i].age<<"\t";
if(staff[i].category==1)
cout<<"Academic"<<"\t";
else if(staff[i].category==2)
cout<<"Non-Academic"<<"\t";
if(staff[i].scheme==1)
cout<<"Pension on 56 years"<<"\t";
else if(staff[i].scheme==2)
cout<<"Pension on 60 years"<<"\t";
cout<<endl;
}
int count1=0,count2=0,count3=0,count4=0;
for(int i=0;i<10;i++)
{
if(staff[i].category==1)
{
if(staff[i].scheme==1)
{
count1++;
}
else if(staff[i].scheme==2)
{
count2++;
}
}
else if(staff[i].category==2)
{
if(staff[i].scheme==1)
{
count3++;
}
else if(staff[i].scheme==2)
{
count4++;
}
}
}
cout<<endl;
cout<<"Report of selected Scheme"<<endl;
cout<<"Category Academic"<<endl;
cout<<"\t\tPension 56 : "<<count1<<endl;
cout<<"\t\tPension 60 : "<<count2<<endl;
cout<<"Report of selected Scheme"<<endl;
cout<<"Category Non-Academic"<<endl;
cout<<"\t\tPension 56 : "<<count3<<endl;
cout<<"\t\tPension 60 : "<<count4<<endl;
return 0;
}

Step by step
Solved in 2 steps

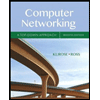
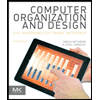
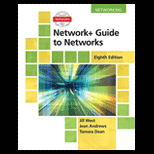
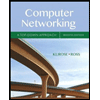
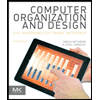
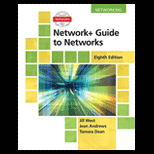
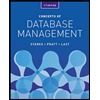
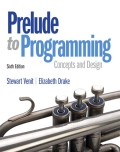
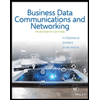