Write a program with a car's miles/gallon and gas dollars/gallon (both doubles) as input, and output the gas cost for 20 miles, 75 miles, and 500 miles. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: System.out.printf("%.2f", yourValue); The output ends with a new line. Ex: If the input is: 20.0 3.1599 the output is: 3.16 11.85 79.00
Driving is expensive. Write a program with a car's miles/gallon and gas dollars/gallon (both doubles) as input, and output the gas cost for 20 miles, 75 miles, and 500 miles.
Output each floating-point value with two digits after the decimal point, which can be achieved as follows:
System.out.printf("%.2f", yourValue);
The output ends with a new line.
Ex: If the input is:
20.0 3.1599
the output is:
3.16 11.85 79.00
For some reason my code is not functioning for all inputs to display the requested outputs.
this is my code used:
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
double n1 = sc.nextDouble();
double n2 = sc.nextDouble();
double product1 = n2*20/20;
double product2 = n2*75/20;
double product3 = n2*500/20;
System.out.printf("%.2f %.2f %.2f",product1,product2,product3);
System.out.println();
}
}
this one is showing correctly:

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

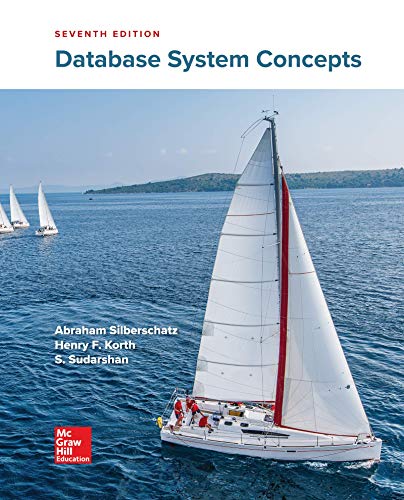
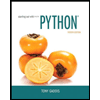
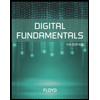
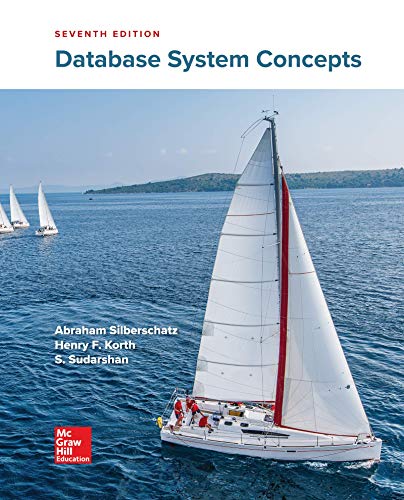
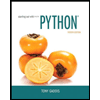
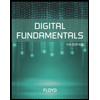
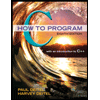
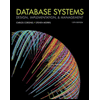
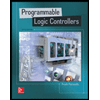