Write a program using c++ to implement a character-based Binary Search Tree (BST). Each node in the BST should be store student name (string) as follows: class Node{// node prototype public: char ch;// this letter is taken from student first letter string studentName; Node *leftChild; Node *rightChild; }; For example, if a student’s name is Adam then ch will be equals to A. If the student’s name is Lee then ch will be equal to L. The insertion of a node to the BST is based on the first letter of the student name. Your program should display the following: 1. Insert a node (base on ch) 2. Find a node (base on student name) 3. Delete a node (consider all three cases of delete) 4. Exit
- Write a program using c++ to implement a character-based Binary Search Tree (BST). Each node in the BST should be store student name (string) as follows:
class Node{// node prototype
public:
char ch;// this letter is taken from student first letter
string studentName;
Node *leftChild;
Node *rightChild;
};
For example, if a student’s name is Adam then ch will be equals to A. If the student’s name is Lee then ch will be equal to L.
The insertion of a node to the BST is based on the first letter of the student name.
Your program should display the following:
1. Insert a node (base on ch) 2. Find a node (base on student name) 3. Delete a node (consider all three cases of delete) 4. Exit
|
Step by step output:
------------------------------------------------------------------------------------------------------------
- Insert a node (base on ch)
- Find a node (base on student name)
- Delete a node (consider all three cases of delete)
- Exit
in the picture
and Output/results provided and explain the



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

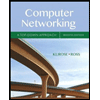
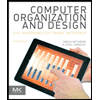
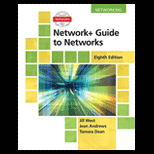
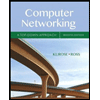
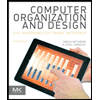
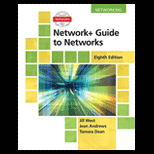
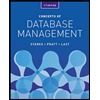
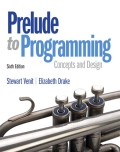
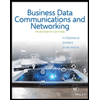