Write a program, using a function, that will check a single integer to determine if it is prime number or not.


A number is said to be prime number if it has only two factors 1 and itself
#include <iostream>
using namespace std;
bool isPrime(int n){
int factors=0; //variable to store number of factors
for(int i=1;i<=n;i++){ //i from 1 to n
if(n%i==0) //if i exactly divides n
factors++; //i is factor of n so increment factors by 1
}
if(factors==2) //if factors is 2
return true; //number is prime and return true
else //if factors is not 2
return false; //number is not prime and return false
}
int main()
{
int x;
cout<<"Enter a positve integer enter to check: ";
cin >> x; //input a number
if(isPrime(x)){ //call the function and it returns true
cout << x << " is a prime number"; //print message saying number is prime
}
else{ //if function call returned false
cout << x << " is not a prime number"; //print a message saying number is not prime
}
return 0;
}
Step by step
Solved in 3 steps with 1 images

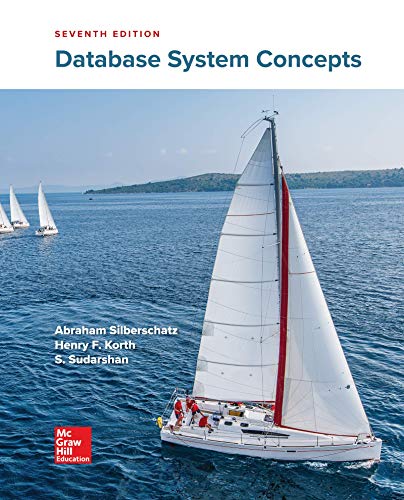
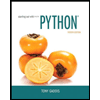
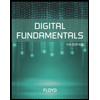
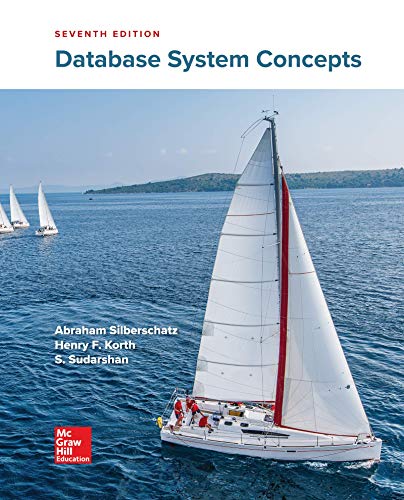
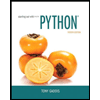
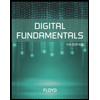
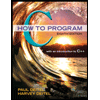
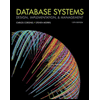
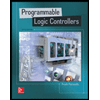