Write a program to provide information on the height of a ball thrown straight up into the air. The program should request as input the initial height, h feet, and the initial velocity, v feet per second. The height of the ball after t seconds is, in feet: h + vt – 16t2 --


def getInput():
# The above line
# we define a fucntion
# of name getInput()
hei = int(input("The starting(initial) height of the ball Please provide:"))
# input of integer from the User
# and stored in hei which is height
vel = int(input("The starting(initial) velocity of the ball Please provide :"))
# input of integer from the User
# and stored in vel which is velocity
isValid(hei,vel)
# The above is we called isValid() function
# With two arguments like hei and vel
# velocity and height repectively
def isValid(hei,vel):
# The above is we define a isValid() function
# With two arguments like hei and vel
# velocity and height repectively which is passed by
# isValid(hei, vel) fucntion
if(hei<=0):
# here we are
# checking the condition
# as hei which is height is <= 0
# condition matches then
# the below line is executed
print("Please provide Height which should be a positive number.")
elif(vel<=0):
# here we are
# checking the condition
# as vel which is velocity is <= 0
# condition matches then
# the below line is executed
print("Please provide Velocity which should be a positive number.")
else:
# here we are
# executing this block
# if none of the above two
# condition matches then
# the below line is executed
height = maximumHeight(hei,vel)
# The above line called
# maximumHeight fucntion
# with two parameter passsing and
# stored the result which
# is return by maximumHeight function
print("The maximum height of the ball is",height," feet.")
# the above line display
# the return the value by maximumHeight function
time = approxTime(hei,vel)
# The above line called
# the approxTime with
# two parameters passing and
# stored the result on time which
# is return by the approxTime
print("The ball will hit the ground after approximately ",round(time,2)," seconds.")
# the above line display which is
# the return the value by approxTime function
def maximumHeight(hei,vel):
# This above line we
# define the maximumHeight function
# with two arguments which is passed through
# called function
t = vel/32;
# The above line we divide
# velocity/32 to get the time
maxHeight = hei + (vel*t) - (16 * (t*t))
# the above line
# calulated the maxHeight
# with the formula hei + (vel*t) - (16 * (t*t))
return maxHeight
# this will return the value
# to the called fucntion
def approxTime(hei,vel):
# here in the above line we
# define the approxTime function with
# two arguments of
# hei and vel height and velocity respectively
t=0
# the above line we initialize
# time with 0 as t = 0
height=hei+(vel*t)-16*(t*t)
# here in the above line
# we calculate
# height with the height formula as
# hei+(vel*t)-16*(t*t) and stored in hei
while height>=0:
# the above loop we define
# which executes
# until condtion matched
t+=.01
# the above line
# we added the time(t) with time(t)
# and store in time (t)
height=hei+(vel*t)-16*(t*t)
# here we calculate
# height as with
# the formula height=hei+(vel*t)-16*(t*t)
# stored the resutl in height
# if height is >= 0
# loop will break
return t
#this line of code
# will retrun the reuslt to the
# called function
getInput()
# here in the above line we called the
# getIbput() function
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

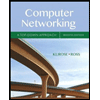
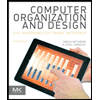
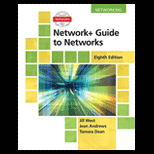
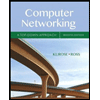
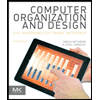
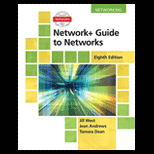
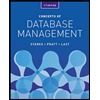
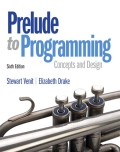
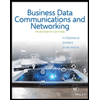