Write a program to provide information on the height of a ball thrown straight up into the air. The program should request as input the initial neight, h feet, and the initial velocity, v feet per second. The height of the
Write a program to provide information on the height of a ball thrown straight up into the air. The program should request as input the initial neight, h feet, and the initial velocity, v feet per second. The height of the
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
How could I modify this program so that I can include the try statement with the except clause to make sure that the user uses number and not a character? Comment that part out too with explanation please

Transcribed Image Text:OID 习t小i=a O
1+v→<印印
O def getInput (): #Defined the getInput function
h=int (input("Enter the initial height of the ball:")) #Prompts user to enter a integer for the initial height of the ball and stores it in h which
v=int(input("Enter the initial velocity of the ball:"))#Prompts user to enter a integer for the initial velocity of the ball and stores it in v whi
isValid(h,v)#Called isValid() function with two arguments h and v
20
def isvalid(h,v): #Defined the isValid function with two arguments like h and v
if(h<=0): #Checks the condition. If height is <=0, the condition matches and the below line is executed
print("Make sure your velocity and height is a positive number") #Disploys not a positive number if height and velocity is (=0
else: #Execute this block if the condition above doesn'
height= maximumHeight(h,v) #Execute this Line if the condition doesn't metch. Called maximumHeight function with two parameters h and v and stor
print("The maximum height of the ball is", height,"feet.") #Displayed and returns the value by maximumHeight function
time=approxTime (h, v)#Called approxTime with two parameters passing and stored the result which is returned by the approxTime
print("The ball wili hit the ground after approximately", round (time, 2, "beconds.") #Displays the returned value from the approxTime function
match
E def maximumHeight (h, v): #Defined maximumHeight function with two arguments which is passed through called functions
t=(v/32) #Divide velocity by 32 to get the time
maxHeight= h+(v*t)-(16 (t*t)) #calculates the maxtleight with the formula
return maxHeight #return the value to the called function
4E def approxTime(h, v): #Defined approxTime function with two arguments ofh and v
t=0 #Initiolize time with e
height=h+(v*t)-16*(t*t) #calculate height with the height formula and stored in height
while height >=0: #Loop defined executes until condition is matched
+= 0.01 fadded time(t) with time(t) and stored in time(t)
height=h+(v*t)-16* (t*t) #calculate height with the formula and stores the result in height. If height is >=0, Loop wilL break
return t
getInput () #Calls the getInput () function
Program Quiz 2.py x
1:5
pall:34
23.0625 feet.
approximately 2 seconds.
ed
11:5
hall:34
%23

Transcribed Image Text:Write a program to provide information on the height of a ball thrown
straight up into the air. The program should request as input the initial
height, h feet, and the initial velocity, v feet per second. The height of the
ball after t seconds is, in feet:
h+ vt – 161²
The program should perform the following two calculations:
1. Determine the maximum height of the ball. Note: The ball will reach its
maximum height after v/32 seconds.
2. Determine the approximately when the ball will hit the ground. Hint:
Calculate the height after every 0.01 second and determine when the
height is not longer a positive number.
A function named getlnput should be used to obtain the values of h and v
and that function should call a function named isValid to ensure that the
input values are positive numbers. Each of the tasks 1 and 2 should be
carried out by functions. Also within one of your function you must use
the return feature (you can use this feature in all functions if you want as
well). Below is example of the inputs and outputs that you could get:
Enter the initial height of the ball: 5
Enter the initial velocity of the ball: 34
The maximum height of the ball is 23.06 feet.
The ball will hit the ground after approximately 2.27 seconds.
Expert Solution

Step 1
def main():
h = 0
v = 0
getInput(h, v)
def getInput(h, v):
h = eval(input("Enter the initial height of the ball: "))
v = eval(input("Enter the initial velocity of the ball: "))
isValid(h,v)
def isValid(h,v):
if ((h<= 0) or (v <= 0)):
print("Please enter positive values")
getInput(h,v)
else:
maxHeight(h,v)
def maxHeight(h,v):
t = (v/32)
maxH = (h + (v*h) - (16*t*t))
print("The maximum height of the ball is", maxH, "feet.")
ballTime(h,v)
def ballTime(h,v):
ballHeight = (h + (v*t) - (16*t*t))
while (ballHeight >= 0):
t += 0.1
print("The ball will hit the ground after approximately", t, "seconds.")
main()
Step by step
Solved in 2 steps

Recommended textbooks for you
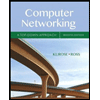
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
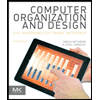
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
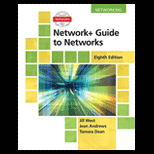
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
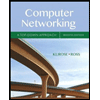
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
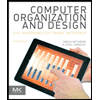
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
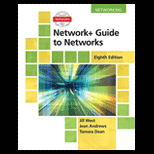
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
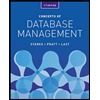
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
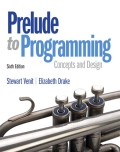
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
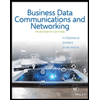
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY