Write a program that will ask the user to enter the amount of purchase. # The program should then compute the state and county sales tax. Assume # the state sales tax is 5 percent and the county sales tax is 2.5 percent. # The program should display the amount of purchase, the state sales tax, # the county sales tax, the total sales tax, and the total of the sale # (which is the sum of the amount of purchase plus the total sales tax). # Hint: Use the value of 0.025 to represent 2.5 percent, and 0.05 to # represent 5 percent. # ***************************************************************************** # constants are variables that always have the same value, within the program. The convention is to use # all caps to define these variables. For example: # INTEREST_RATE = 0.2 # Note: You must use STATE_TAX_RATE and COUNTY_TAX_RATE named constants, # amount_purch, state_sales_tax, county_sales_tax, total_sales_tax and # total_order_amount variables. The input and output must have appropriate # labels such as "Enter purchase amount:" or "Total order amount is", with # the resulting numbers formatted as currency with two decimals showing a # thousand separator. # # ***************************************************************************** # Test: Your input and output should look like this: # Enter the purchase amount: 1234.45 # Summary of the order # Amount purchased is $1,234.45 # State sales tax is $61.72 # County sales tax is $30.86 # Total sales tax is $92.58 # Total order amount is $1,327.03 # ***************************************************************************** # Get the amount of purchase # Set the state and county tax rate constants # Calculate the state and county taxes on the purchase # Calculate the total sales tax and total order amount # Display the results print('Summary of the order')
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Write a
# The program should then compute the state and county sales tax. Assume
# the state sales tax is 5 percent and the county sales tax is 2.5 percent.
# The program should display the amount of purchase, the state sales tax,
# the county sales tax, the total sales tax, and the total of the sale
# (which is the sum of the amount of purchase plus the total sales tax).
# Hint: Use the value of 0.025 to represent 2.5 percent, and 0.05 to
# represent 5 percent.
# *****************************************************************************
# constants are variables that always have the same value, within the program. The
convention is to use
# all caps to define these variables. For example:
# INTEREST_RATE = 0.2
# Note: You must use STATE_TAX_RATE and COUNTY_TAX_RATE named constants,
# amount_purch, state_sales_tax, county_sales_tax, total_sales_tax and
# total_order_amount variables. The input and output must have appropriate
# labels such as "Enter purchase amount:" or "Total order amount is", with
# the resulting numbers formatted as currency with two decimals showing a
# thousand separator.
#
# *****************************************************************************
# Test: Your input and output should look like this:
# Enter the purchase amount: 1234.45
# Summary of the order
# Amount purchased is $1,234.45
# State sales tax is $61.72
# County sales tax is $30.86
# Total sales tax is $92.58
# Total order amount is $1,327.03
# *****************************************************************************
# Get the amount of purchase
# Set the state and county tax rate constants
# Calculate the state and county taxes on the purchase
# Calculate the total sales tax and total order amount
# Display the results
print('Summary of the order')

The following code is a C++ program that calculates the state and county sales tax for a given amount of purchase. The user is prompted to enter the amount of purchase and the program uses this value to calculate the state sales tax (5%) and the county sales tax (2.5%). The program then calculates the total sales tax (sum of the state and county sales tax) and the total order amount (sum of the amount of purchase and the total sales tax). Finally, the program displays the results including the amount of purchase, state and county sales tax, total sales tax, and total order amount, formatted as currency with two decimal places and a thousands separator. The program uses the cin stream to get the amount of purchase from the user, the cout stream to display the results, and the fixed and setprecision manipulators to format the numbers.
Step by step
Solved in 3 steps with 1 images

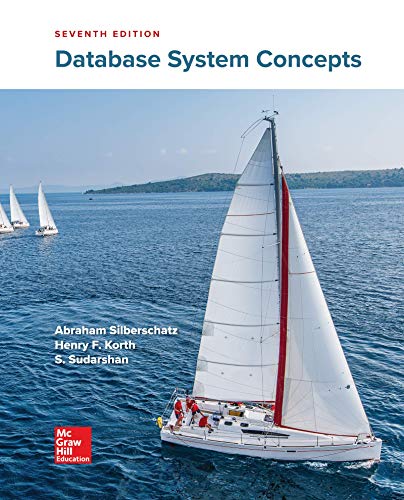
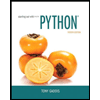
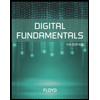
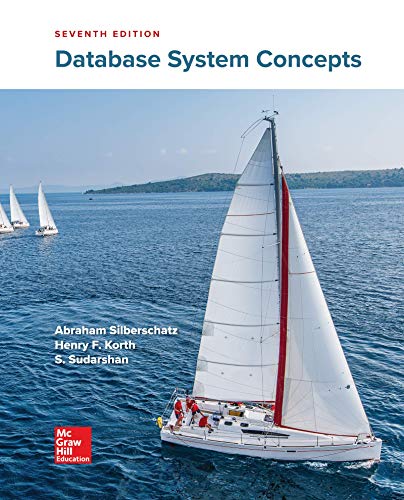
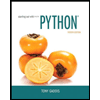
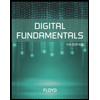
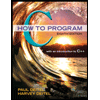
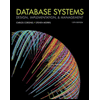
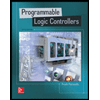