Write a program that uses UNIX system calls to “ping-pong” a byte between two processes over a pair of pipes, one for each direction. Measure the program’s performance, in exchanges per second.
Write a program that uses UNIX system calls to “ping-pong” a byte between two processes over a pair of pipes, one for each direction. Measure the program’s performance, in exchanges per second.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a
a pair of pipes, one for each direction. Measure the program’s performance, in exchanges per
second.
![Transcription for Educational Website:
---
**2. Answer Memory Allocation Question**
```c
void func() {
int x; // declares an integer on the stack
...
}
void func() {
int *x = (int *) malloc(sizeof(int));
...
}
#include <stdlib.h>
...
void *malloc(size_t size);
double *d = (double *) malloc(sizeof(double));
int *x = malloc(10 * sizeof(int));
printf("%d\n", sizeof(x));
int x[10];
printf("%d\n", sizeof(x));
```
*Note: Allocating and freeing memory is fraught with possible errors.*
**Can you explain?**
See text section 14.4 for important caveats.
---
In this example, the focus is on understanding memory allocation in C programming, specifically comparing stack and heap allocation and their implications:
- The first code block shows an integer variable `x` declared on the stack.
- The second block demonstrates dynamic memory allocation using `malloc`, which allocates memory on the heap.
- The importance of including the `<stdlib.h>` library, which provides the `malloc` function, is highlighted.
- An example of allocating memory for a `double` variable using `malloc` is provided.
- The size of dynamically and statically allocated integer arrays is printed, illustrating the difference between stack and heap memory sizes.
- The note highlights that correctly handling memory allocation and deallocation is critical to prevent errors.
For a detailed explanation, refer to text section 14.4.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F37d58292-49ac-4512-b8fc-4d8dd687eb61%2F7aec751e-e552-4cf1-94cb-f8d2329dc488%2Fzdklo6d_processed.png&w=3840&q=75)
Transcribed Image Text:Transcription for Educational Website:
---
**2. Answer Memory Allocation Question**
```c
void func() {
int x; // declares an integer on the stack
...
}
void func() {
int *x = (int *) malloc(sizeof(int));
...
}
#include <stdlib.h>
...
void *malloc(size_t size);
double *d = (double *) malloc(sizeof(double));
int *x = malloc(10 * sizeof(int));
printf("%d\n", sizeof(x));
int x[10];
printf("%d\n", sizeof(x));
```
*Note: Allocating and freeing memory is fraught with possible errors.*
**Can you explain?**
See text section 14.4 for important caveats.
---
In this example, the focus is on understanding memory allocation in C programming, specifically comparing stack and heap allocation and their implications:
- The first code block shows an integer variable `x` declared on the stack.
- The second block demonstrates dynamic memory allocation using `malloc`, which allocates memory on the heap.
- The importance of including the `<stdlib.h>` library, which provides the `malloc` function, is highlighted.
- An example of allocating memory for a `double` variable using `malloc` is provided.
- The size of dynamically and statically allocated integer arrays is printed, illustrating the difference between stack and heap memory sizes.
- The note highlights that correctly handling memory allocation and deallocation is critical to prevent errors.
For a detailed explanation, refer to text section 14.4.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
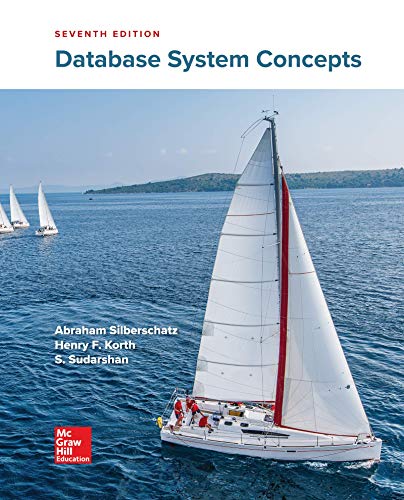
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
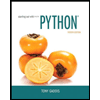
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
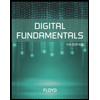
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
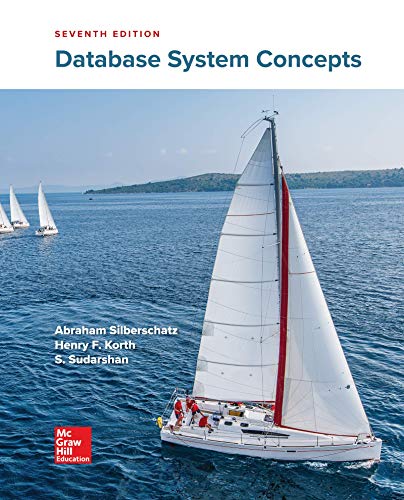
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
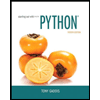
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
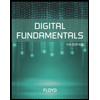
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
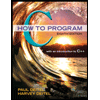
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
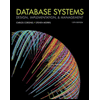
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
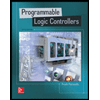
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education