Write a program that takes in a positive integer as input, and outputs a string of 1's and O's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2 Note: The above algorithm outputs the O's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: the output is: 110 Your program must define and call the following two methods. The method integerToReverseBinary() should return a string of 1's and O's representing the integer in binary (in reverse). The method reverseString() should return a string representing the input string in reverse. public static String integerTOReverseBinary (int integerValue) public static String reverseString(String inputString) Note: This is a lab from a previous chapter that now requires the use of a method.
Write a program that takes in a positive integer as input, and outputs a string of 1's and O's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2 Note: The above algorithm outputs the O's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: the output is: 110 Your program must define and call the following two methods. The method integerToReverseBinary() should return a string of 1's and O's representing the integer in binary (in reverse). The method reverseString() should return a string representing the input string in reverse. public static String integerTOReverseBinary (int integerValue) public static String reverseString(String inputString) Note: This is a lab from a previous chapter that now requires the use of a method.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The
![```java
import java.util.Scanner;
public class LabProgram {
/* Define your method here */
public static void main(String[] args) {
/* Type your code here. */
}
}
```
### Explanation:
This Java code provides a basic structure for a program named `LabProgram`. It includes:
- **Import Statement**:
- `import java.util.Scanner;`
- This line imports the `Scanner` class from the `java.util` package, which is used to capture user input.
- **Class Definition**:
- `public class LabProgram`
- This line defines a public class named `LabProgram`. In Java, every application must contain at least one class definition. Here, the class is named `LabProgram`.
- **Method Placeholder**:
- `/* Define your method here */`
- This comment serves as a placeholder where you can define additional methods within the class.
- **Main Method**:
- `public static void main(String[] args)`
- This is the main method, the entry point for any Java program. The `String[] args` parameter is used for command-line arguments.
- **Main Method Placeholder**:
- `/* Type your code here. */`
- This comment provides a space to write executable code within the `main` method.
This template is commonly used as a starting point for Java programs, allowing programmers to structure their code and add functionalities as needed.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8fe79bd2-2df3-45cc-abc0-ffa837cca5d8%2F3315c3aa-1cbe-4522-a294-0727389d5a2f%2Fagdg7fj_processed.png&w=3840&q=75)
Transcribed Image Text:```java
import java.util.Scanner;
public class LabProgram {
/* Define your method here */
public static void main(String[] args) {
/* Type your code here. */
}
}
```
### Explanation:
This Java code provides a basic structure for a program named `LabProgram`. It includes:
- **Import Statement**:
- `import java.util.Scanner;`
- This line imports the `Scanner` class from the `java.util` package, which is used to capture user input.
- **Class Definition**:
- `public class LabProgram`
- This line defines a public class named `LabProgram`. In Java, every application must contain at least one class definition. Here, the class is named `LabProgram`.
- **Method Placeholder**:
- `/* Define your method here */`
- This comment serves as a placeholder where you can define additional methods within the class.
- **Main Method**:
- `public static void main(String[] args)`
- This is the main method, the entry point for any Java program. The `String[] args` parameter is used for command-line arguments.
- **Main Method Placeholder**:
- `/* Type your code here. */`
- This comment provides a space to write executable code within the `main` method.
This template is commonly used as a starting point for Java programs, allowing programmers to structure their code and add functionalities as needed.

Transcribed Image Text:**Binary Conversion Program**
Write a program that takes in a positive integer as input and outputs a string of 1's and 0's representing the integer in binary. For an integer `x`, the algorithm is:
```
As long as x is greater than 0
Output x % 2 (remainder is either 0 or 1)
x = x / 2
```
**Note:** The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string.
**Example:**
If the input is:
```
6
```
the output is:
```
110
```
Your program must define and call the following two methods. The method `integerToReverseBinary()` should return a string of 1's and 0's representing the integer in binary (in reverse). The method `reverseString()` should return a string representing the input string in reverse.
```java
public static String integerToReverseBinary(int integerValue)
public static String reverseString(String inputString)
```
**Note:** This is a lab from a previous chapter that now requires the use of a method.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
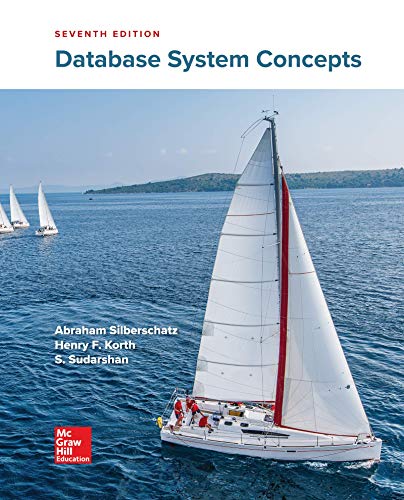
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
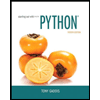
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
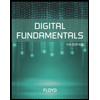
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
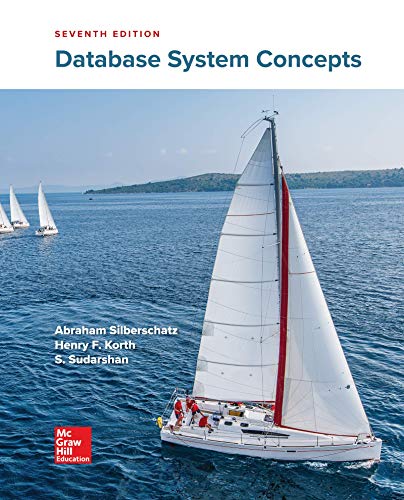
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
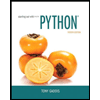
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
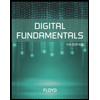
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
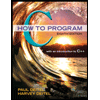
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
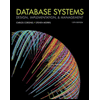
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
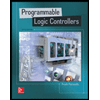
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education