Write a program that takes as input an arithmetic expression followed by a semicolon ;. The program outputs whether the expression contains matching grouping symbols. For example, the arithmetic expressions {25 + (3 – 6) * 8} and 7 + 8 * 2 contains matching grouping symbols. However, the expression 5 + {(13 + 7) / 8 - 2 * 9 does not contain matching grouping symbols. If the expression contains matching grouping symbols, the program output should contain the following text: Expression has matching grouping symbol If the expression does not contain matching grouping symbols, the program output should contain the following text: Expression does not have matching grouping symbo
Write a program that takes as input an arithmetic expression followed by a semicolon ;. The program outputs whether the expression contains matching grouping symbols. For example, the arithmetic expressions {25 + (3 – 6) * 8} and 7 + 8 * 2 contains matching grouping symbols. However, the expression 5 + {(13 + 7) / 8 - 2 * 9 does not contain matching grouping symbols. If the expression contains matching grouping symbols, the program output should contain the following text: Expression has matching grouping symbol If the expression does not contain matching grouping symbols, the program output should contain the following text: Expression does not have matching grouping symbo
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a
The program outputs whether the expression contains matching grouping symbols.
For example, the arithmetic expressions {25 + (3 – 6) * 8} and 7 + 8 * 2 contains matching grouping symbols.
However, the expression 5 + {(13 + 7) / 8 - 2 * 9 does not contain matching grouping symbols.
If the expression contains matching grouping symbols, the program output should contain the following text:
Expression has matching grouping symbol
If the expression does not contain matching grouping symbols, the program output should contain the following text:
Expression does not have matching grouping symbols.

Transcribed Image Text:Chrome
File
Edit
View
History
Bookmarks
Реople
Tab
Window
Help
77%
Fri 9:24 AM
MindTap - Cengage Learning
MindTap - Cengage Learning
X G how to screenshot on macbook x +
ng.cengage.com/static/nb/ui/evo/index.html?elSBN=9780357425282&id=990036018&snapshotld=1867908&
Реace v
«
CENGAGE MINDTAP
Q Search this course
Му Home
Midterm A
Courses
main.cpp
myStack.h
stackADT.h
>- Terminal
+
3 #ifndef H_StackType
O Catalog and Study Tools
4 #define H_StackType
5
А-Z
Partner Offers
6 #include <iostream>
7 #include <cassert>
Rental Options
</>
8
College Success Tips
9 #include "stackADT.h"
10
Career Success Tips
11 using namespace std;
12
RECOMMENDED FOR YOU
13 template <class Type>
14 class stackType: public stackADT<Type>
15 {
16 public:
17
const stackType<Type>& operator=(const stackType<Type>&);
18
void initializeStack();
Staying Safe on Social
19
bool isEmptyStack() const;
Networks
20
bool isFullstack() const;
21
void push(const Type& newItem);
Type top() const;
void pop();
stackType (int stackSize
22
О Нelp
23
Give Feedback
24
100);
stackType(const stackType<Type>& otherStack);
~stackType();
25
26
27
28
20 privato!
國T山
454
ОСТ
10
3
16
Xd
Ai Ps
(3D)
HD
!!

Transcribed Image Text:Chrome
File
Edit
View
History
Bookmarks
Реople
Tab
Window
Help
79%
Fri 9:22 AM
MindTap - Cengage Learning
MindTap - Cengage Learning
X G how to screenshot on macbook x +
ng.cengage.com/static/nb/ui/evo/index.html?elSBN=9780357425282&id=990036018&snapshotld=1867908&
Реace v
«
CENGAGE MINDTAP
Q Search this course
Му Home
Midterm A
i
Courses
main.cpp
|myStack.h
stackADT.h
>- Terminal
+
1 //Header file: stackADT.h
O Catalog and Study Tools
2
3 #ifndef H_StackADT
А-Z
Partner Offers
4 #define H_StackADT
Rental Options
5
6 template <class Type>
7 class stackADT
8 {
College Success Tips
Career Success Tips
9 public:
10
virtual void initializeStack() = 0;
%3D
RECOMMENDED FOR YOU
11
virtual bool isEmptyStack() const = 0;
12
virtual bool isFullStack() const
0;
%3D
13
virtual void push(const Type& newItem)
0;
virtual Type top() const
virtual void pop() = 0;
14
0;
%3D
15
16
Staying Safe on Social
17 };
Networks
18
19 #endif
О Нelp
20
Give Feedback
:國T山
454
ОСТ
10
3
16
Xd
Ai Ps
(3D
HD
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
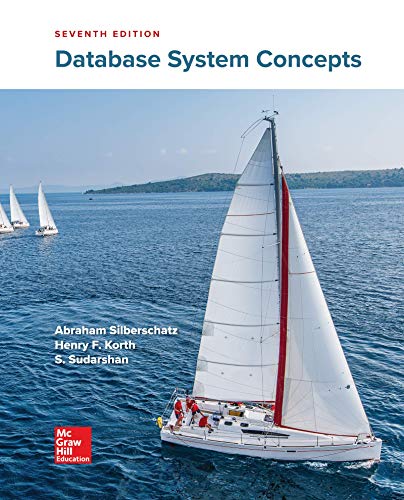
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
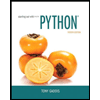
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
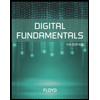
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
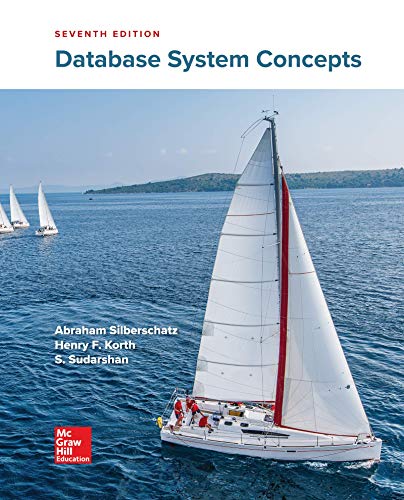
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
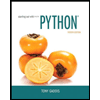
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
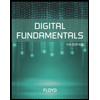
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
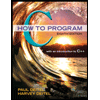
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
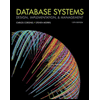
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
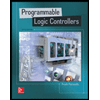
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education