Write a program that takes a list of S integers and performs the following functions. Create two functions, and each function takes a single parameter my_list, which is a Python list: delete_prime(my_list) - returns a list with all prime numbers removed. The function returns a new list, and the parameter input my_list is not modified. Input: list Return value: list reverse_list(my_list) - reverses my_list. The parameter input my_list is modified. Input: list Return value: None Notes: Since a list is a mutable object, you may need to make a copy of the original list to pass as input parameter to the function. Modern mathematicians define a number as prime if it is divided by exactly two numbers. To keep things simple for this exercise, assume all elements of the list have a value greater than 1. Constraint: You are not allowed to use my_list[::-1], my_list.reverse(), or reversed(my_list). Input Format Assume the inputs are integers. The first line contains the integer S where S is the number of elements in the list. The next S lines each contain an integer X. These are the elements in the list. Constraints The input list has at least 2 elements and a maximum of 30 elements: 2 <= S <= 30 Each element X in the list is between 2 and 100: 2 <= X <= 100 Assume no invalid inputs are given. No text will be given as input, no negative integers, and there are enough inputs needed for the program to function correctly. **You are not allowed to use my_list[::-1], my_list.reverse(), or reversed(my_list). ** Output Format The output is two lists of integers - one with the prime numbers removed and the other the reverse of the original list. SEE SAMPLE INPUTS AND OUTPUTS BELOW
HELP PYTHON
Write a
delete_prime(my_list) - returns a list with all prime numbers removed. The function returns a new list, and the parameter input my_list is not modified.
Input: listreverse_list(my_list) - reverses my_list. The parameter input my_list is modified.
Input: listNotes:
- Since a list is a mutable object, you may need to make a copy of the original list to pass as input parameter to the function.
- Modern mathematicians define a number as prime if it is divided by exactly two numbers. To keep things simple for this exercise, assume all elements of the list have a value greater than 1.
- Constraint: You are not allowed to use my_list[::-1], my_list.reverse(), or reversed(my_list).
Input Format
Assume the inputs are integers.
The first line contains the integer S where S is the number of elements in the list. The next S lines each contain an integer X. These are the elements in the list.
Constraints
The input list has at least 2 elements and a maximum of 30 elements: 2 <= S <= 30 Each element X in the list is between 2 and 100: 2 <= X <= 100
Assume no invalid inputs are given. No text will be given as input, no negative integers, and there are enough inputs needed for the program to function correctly.
**You are not allowed to use my_list[::-1], my_list.reverse(), or reversed(my_list). **
Output Format
The output is two lists of integers - one with the prime numbers removed and the other the reverse of the original list.
SEE SAMPLE INPUTS AND OUTPUTS BELOW
![Sample Input 0
7
4
7
10
3
90
6.
Sample Output 0
Prime Removed:
[4, 10, 90, 6]
Reversed:
[2, 6, 90, 3, 10, 7, 4]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Feb6e5c38-a555-445d-a8a8-e8c0b1acef8f%2F92095494-66f1-40ac-a571-1add65cddf0f%2Fk4b5so_processed.png&w=3840&q=75)
![Sample Input 1
10
19
22
3
28
26
17
18
4
28
100
Sample Output 1
Prime Removed:
[22, 28, 26, 18, 4, 28, 100]
Reversed:
[100, 28, 4, 18, 17, 26, 28, 3, 22, 19]](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Feb6e5c38-a555-445d-a8a8-e8c0b1acef8f%2F92095494-66f1-40ac-a571-1add65cddf0f%2Fjuumve_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

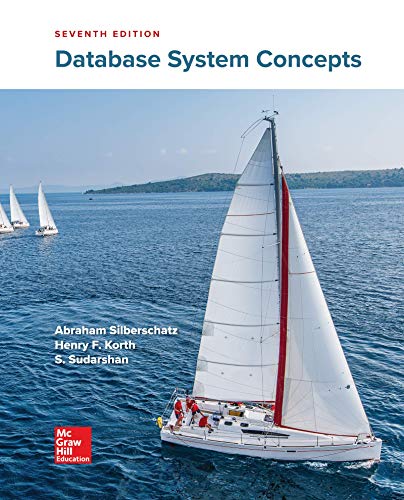
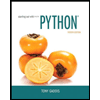
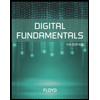
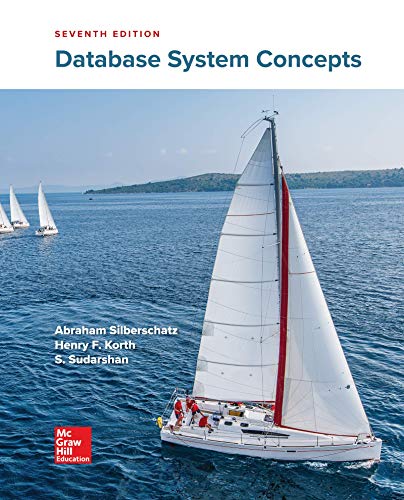
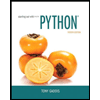
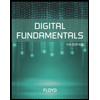
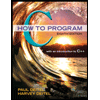
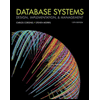
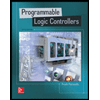