Write a program that reads two lists of integers from input into two arrays and outputs the sum of multiplying the corresponding list items. The program first reads an integer representing the length of each list, followed by two lists of integers. Ex: If the input is: 3 1 2 3 3 2 1 the program calculates (1 * 3) + (2*2)+(3 * 1) and outputs 10 Ex: If the input is: 4 2 3 4 5 1 1 1 1 the program calculates (2* 1) + (3 * 1) + (4 * 1) + (5 * 1) and outputs 14
Write a program that reads two lists of integers from input into two arrays and outputs the sum of multiplying the corresponding list items. The program first reads an integer representing the length of each list, followed by two lists of integers. Ex: If the input is: 3 1 2 3 3 2 1 the program calculates (1 * 3) + (2*2)+(3 * 1) and outputs 10 Ex: If the input is: 4 2 3 4 5 1 1 1 1 the program calculates (2* 1) + (3 * 1) + (4 * 1) + (5 * 1) and outputs 14
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In Java please.

Transcribed Image Text:Write a program that reads two lists of integers from input into two arrays and outputs the sum of multiplying the corresponding list items.
The program first reads an integer representing the length of each list, followed by two lists of integers.
Ex: If the input is:
3
1 2 3
3 2 1
the program calculates (1 * 3) + (2 * 2) + (3 * 1) and outputs
10
Ex: If the input is:
2 3 4 5
1 1 1 1
the program calculates (2 * 1) + (3 * 1) + (4 * 1) + (5 * 1) and outputs
14
![1 import java.util.Scanner;
2
3 public class LabProgram {
4
5
6
7
8
9
10
11
12
13
14}
public static void main(String[] args) {
}
Scanner scnr new Scanner(System.in);
int size;
size = scnr.nextInt();
int[] listA new int[size];
int[] listB
new int[size];
here. */
=
/* Type your code
Develop mode Submit mode
// List A
// List B
Run your program as often as you'd like, before submitting for grading. Below, type any needed
input values in the first box, then click Run program and observe the program's output in the
second box.
Enter program input (optional)
If your code requires input values, provide them here.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb4d4e6a3-fd83-4270-b000-89a89a72868b%2F083d839d-65c2-4a13-91b1-707e2105e135%2Fhsavsrx_processed.png&w=3840&q=75)
Transcribed Image Text:1 import java.util.Scanner;
2
3 public class LabProgram {
4
5
6
7
8
9
10
11
12
13
14}
public static void main(String[] args) {
}
Scanner scnr new Scanner(System.in);
int size;
size = scnr.nextInt();
int[] listA new int[size];
int[] listB
new int[size];
here. */
=
/* Type your code
Develop mode Submit mode
// List A
// List B
Run your program as often as you'd like, before submitting for grading. Below, type any needed
input values in the first box, then click Run program and observe the program's output in the
second box.
Enter program input (optional)
If your code requires input values, provide them here.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
How do i fix this code
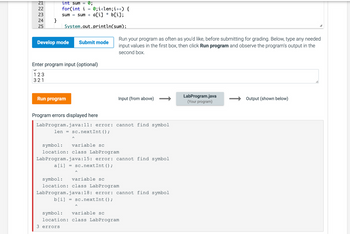
Transcribed Image Text:This image illustrates an error encountered in a Java program due to the misuse of the `Scanner` class. The section at the top shows the Java code, which appears to be working on a mathematical computation involving two arrays `a` and `b`. Here’s a breakdown of each segment:
### Java Code:
- The code initializes variables and inputs data to perform mathematical operations.
- Key sections include the calculation of a `sum`, where each pair of elements from two arrays (`a` and `b`) are multiplied and accumulated.
### Inputs and Errors:
- Users are instructed to enter inputs in the provided box. Example inputs shown are `1 2 3` and `3 2 1`.
- When attempting to run the program, users encounter `Program errors displayed here`:
#### Error Details:
1. **Error Message:**
- `LabProgram.java:11: error: cannot find symbol len = sc.nextInt();`
- **Symbol**: variable `sc`
- **Location**: class `LabProgram`
2. **Repetitive Errors:**
- Similar errors occur at line 15 and line 18, indicating `sc` is used without being properly initialized.
3. **Nature of Errors:**
- The errors suggest that the `Scanner` object `sc` has not been declared. This is essential for capturing user input in Java programs.
- Solution: Declare `Scanner sc = new Scanner(System.in);` at the beginning of the class to resolve these errors.
### Workflow Diagram:
- A workflow diagram illustrates the flow from input gathering to processing through `LabProgram.java` and output generation.
### Conclusion:
These errors need addressing by declaring and initializing the `Scanner` object properly so that inputs can be read and processed without issues. Understanding these common mistakes is crucial for beginners learning Java programming.
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
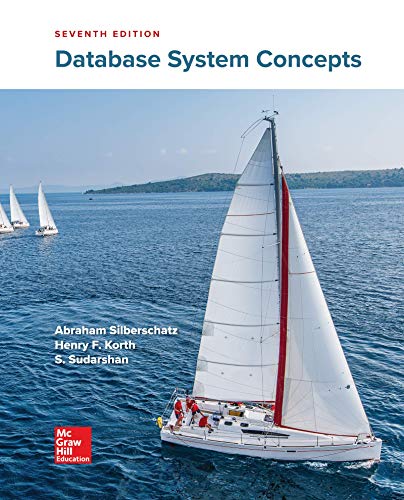
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
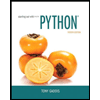
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
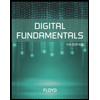
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
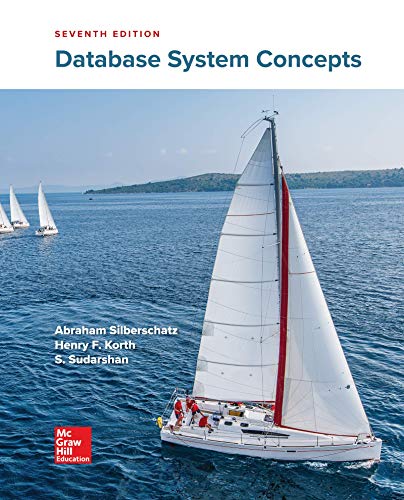
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
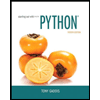
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
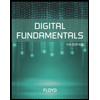
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
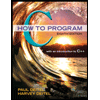
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
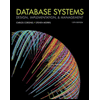
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
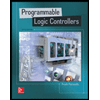
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education