Write a program that reads a list of words. Then, the program outputs those words and their frequencies. The input begins with an integer indicating the number of words that follow. Assume that the list will always contain fewer than 20 words. Ex: If the input is: 5 hey hi Mark hi mark the output is: hey - 1 hi - 2 Mark - 1 hi - 2 mark - 1 Hint: Use two arrays, one array for the strings and one array for the frequencies import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner input = new Scanner(System.in); // Read the number of words int numWords = input.nextInt(); input.nextLine(); // Consume the newline character // Initialize arrays to store words and their frequencies String[] words = new String[numWords]; int[] frequencies = new int[numWords]; // Read and process the words for (int i = 0; i < numWords; i++) { String word = input.next(); boolean found = false; // Check if the word is already in the array for (int j = 0; j < i; j++) { if (word.equalsIgnoreCase(words[j])) { frequencies[j]++; found = true; break; } } // If the word is not found, add it to the arrays if (!found) { words[i] = word; frequencies[i] = 1; } } // Output words and their frequencies for (int i = 0; i < numWords; i++) { if (words[i] != null) { System.out.println(words[i] + " - " + frequencies[i]); } } } }
Write a program that reads a list of words. Then, the program outputs those words and their frequencies. The input begins with an integer indicating the number of words that follow. Assume that the list will always contain fewer than 20 words.
Ex: If the input is:
5 hey hi Mark hi mark
the output is:
hey - 1 hi - 2 Mark - 1 hi - 2 mark - 1
Hint: Use two arrays, one array for the strings and one array for the frequencies
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
// Read the number of words
int numWords = input.nextInt();
input.nextLine(); // Consume the newline character
// Initialize arrays to store words and their frequencies
String[] words = new String[numWords];
int[] frequencies = new int[numWords];
// Read and process the words
for (int i = 0; i < numWords; i++) {
String word = input.next();
boolean found = false;
// Check if the word is already in the array
for (int j = 0; j < i; j++) {
if (word.equalsIgnoreCase(words[j])) {
frequencies[j]++;
found = true;
break;
}
}
// If the word is not found, add it to the arrays
if (!found) {
words[i] = word;
frequencies[i] = 1;
}
}
// Output words and their frequencies
for (int i = 0; i < numWords; i++) {
if (words[i] != null) {
System.out.println(words[i] + " - " + frequencies[i]);
}
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

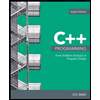
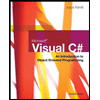
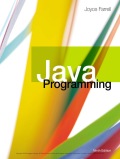
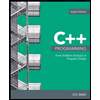
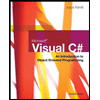
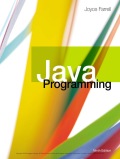