Write a program that prints 20 random numbers between 10 and 99 inclusive. Read in one integer from stdin to use as the seed value.
Write a program that prints 20 random numbers between 10 and 99 inclusive. Read in one integer from stdin to use as the seed value.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:**Loyalty Program Management for Customer Purchases: A Coding Challenge**
This exercise involves revising a program to monitor loyalty points for three customers: A, B, and C. Below are the specifications and an example code snippet to guide you in achieving the program's objectives.
**Program Specifications:**
1. **Input Process:**
- When a customer makes a purchase, the program reads two inputs:
- A string, either "A", "B", or "C", that identifies the customer.
- A float representing the amount spent in dollars.
2. **Program Termination:**
- The program ends when the input reads "nobody", signaling no further purchases.
3. **Loyalty Program Requirements:**
- Customers earn one loyalty point for purchases totaling $10.00 or more.
- If a customer accumulates six loyalty points, their total resets to one point.
- Points are earned and tracked individually, without influence from other customers' purchases.
4. **Code Snippet Overview:**
```cpp
#include <iostream>
using namespace std;
int points = 0;
void updateLoyaltyCard() {
points++;
}
int main() {
int customerAPoints = 0;
int customerBPoints = 0;
int customerCPoints = 0;
string customer;
float purchase;
bool looping = true;
while (looping) {
cout << "Customer: ";
cin >> customer;
if (customer == "nobody") {
break;
}
// Additional code logic will be needed here to manage purchases and points.
}
return 0;
}
```
**Instructions for Code Modification:**
- You can customize the program to effectively fulfill the loyalty program goals mentioned above. This will involve adding logic to calculate and update each customer's points based on the specified conditions.
**Explanation of Code Structure:**
- The program uses a `while` loop to continually prompt for customer actions until "nobody" is entered.
- Variables for each customer (`customerAPoints`, `customerBPoints`, `customerCPoints`) keep track of individual loyalty points.
- Modify the `updateLoyaltyCard()` function and main logic to implement the point earning system and reset condition effectively.
By following the outlined requirements and modifying the code snippet, you will create an effective loyalty program management system for the given customers.

Transcribed Image Text:### Generating Random Numbers in C++
#### Introduction
This tutorial demonstrates how to write a C++ program that generates 20 random numbers between 10 and 99, inclusive. A seed value is read from standard input to initiate the random number generation sequence.
#### Code Explanation
```cpp
#include <iostream>
#include <stdlib.h>
using namespace std;
int main() {
int seed;
cout << "Seed: ";
cin >> seed;
cout << endl;
srand(seed);
return 0;
}
```
#### Detailed Breakdown
- **Header Files**:
- `#include <iostream>`: This allows for input and output operations.
- `#include <stdlib.h>`: Includes functions for performing general functions such as memory allocation, process control, conversions, and more. Specifically, it provides the `srand` function for seeding the random number generator.
- **Namespace**:
- `using namespace std;`: This line allows the use of standard C++ library objects and functions without prefixing them with `std::`.
- **Main Function**:
- `int main() { ... }`: Defines the entry point of the program.
- **Seed Input**:
- `int seed;`: Declares an integer variable, `seed`, to store the user's input.
- `cout << "Seed: ";`: Prompts the user to enter a seed value.
- `cin >> seed;`: Reads the seed value from standard input.
- `cout << endl;`: Outputs a newline character for formatting.
- **Random Number Initialization**:
- `srand(seed);`: Seeds the pseudo-random number generator used by `rand()` with the user-provided seed value, ensuring different sequences of random numbers.
- **Program Exit**:
- `return 0;`: Indicates that the program executed successfully.
This program demonstrates how to set up the random number generator, though does not yet include the logic to generate and print the random numbers themselves. Further logic is required to complete the task, typically involving loops and the `rand()` function to generate and print the numbers.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
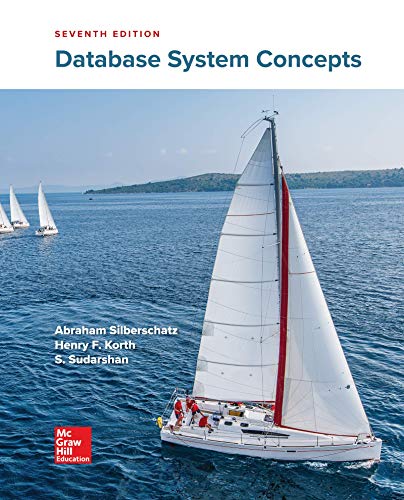
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
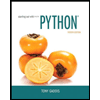
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
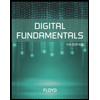
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
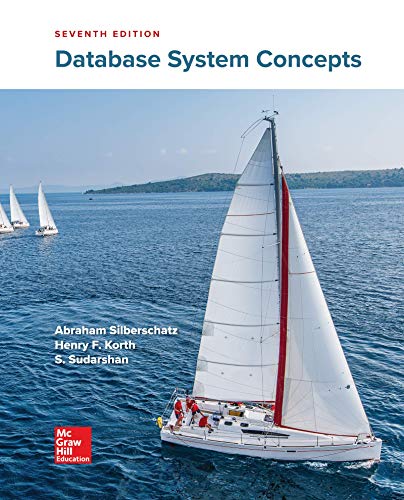
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
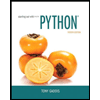
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
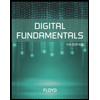
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
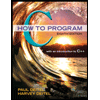
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
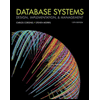
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
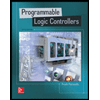
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education