Write a program in C to implement the sequential search algorithm of Chapter 3, Figure 3.1, except that instead of searching a list of telephone numbers for a particular number, have the program search a list of integers for a particular integer. Use the same input mechanism as in the data cleanup programs of Section 9.3.2 to get the list of integers to be searched. Then get the target number to be searched for. The program should output “Successful search, the value is in the list” or “Unsuccessful search, the value is not in the list.” Be sure to test your program for both outcome.
Write a program in C to implement the sequential search algorithm of Chapter 3, Figure 3.1, except that instead of searching a list of telephone numbers for a particular number, have the program search a list of integers for a particular integer. Use the same input mechanism as in the data cleanup programs of Section 9.3.2 to get the list of integers to be searched. Then get the target number to be searched for. The program should output “Successful search, the value is in the list” or “Unsuccessful search, the value is not in the list.” Be sure to test your program for both outcome.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Write a
the program search a list of integers for a particular integer. Use the same input

Transcribed Image Text:**FIGURE 3.1**
1. Get values for **NUMBER**, **n**, **T₁**, ..., **Tₙ**, and **N₁**, ..., **Nₙ**.
2. Set the value of **i** to 1 and set the value of **Found** to NO.
3. While (**Found** = NO) and (**i** ≤ n) do Steps 4 through 7.
4. If **NUMBER** is equal to the i-th number on the list, **T**, then
5. Print the name of the corresponding person, **N_i**.
6. Set the value of **Found** to YES.
Else (**NUMBER** is not equal to **T**)
7. Add 1 to the value of **i**.
8. If (**Found** = NO) then
9. Print the message 'Sorry, this number is not in our directory'.
10. Stop.
**Sequential search algorithm**
---
This diagram provides a step-by-step description of a sequential search algorithm. The algorithm searches for a specific **NUMBER** within a list of numbers, **T₁**, ..., **Tₙ**, and prints the corresponding person's name if the number is found. If the number is not present, it prints an appropriate message. The steps are logically organized to systematically check each entry in the list until a match is found or the list is exhausted.

Transcribed Image Text:### 9.3.2 Data Cleanup (Again)
Now that you’ve seen a bare-bones sample for each language, let’s implement a solution to a considerably more interesting problem. In Chapter 3, we discussed several algorithms to solve the data cleanup problem. In this problem, the input is a set of integer data values (answers to a particular question on a survey, for example) that may contain 0s, although 0s are considered invalid data. The output is to be a clean data set where the 0s have been eliminated. Figure 9.8 is a copy of Figure 3.16. It shows the pseudocode for the converging-pointers data cleanup algorithm, the most time- and space-efficient of the three data cleanup algorithms from Chapter 3.
Our pseudocode does not specify the details of how to “get values.” In the favorite number example, the single input value was entered at the keyboard. The survey data, however, is probably already stored in an electronic file. It might have been collected via an online survey that captured the responses or via paper forms that have been scanned to capture the data in digital form. Designing our programs to read input data from a file, however, is a bit more than we want to get into, so we’ll again assume the input data is typed in at the keyboard.
The pseudocode algorithm of Figure 9.8 is implemented in Ada (Figure 9.9), C++ (Figure 9.10), C# (Figure 9.11), Java (Figure 9.12), and Python (Figure 9.13).
As with the previous, simpler example, you can see that each program follows the outline of the pseudocode algorithm. Each language supports if statements and while loops. The extent of the while loop is denoted by curly braces `{}` in C++ and Java, indentation in Python, and reserved words in Ada.
#### FIGURE 9.8
1. Get values for `n` and the `n` data items
2. Set the value of `legit` to `n`
3. Set the value of `left` to 1
4. Set the value of `right` to `n`
5. **While** `left` is less than `right` do Steps 6 through 10
6. **If** the item at position `left` is not 0 then increase `left
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
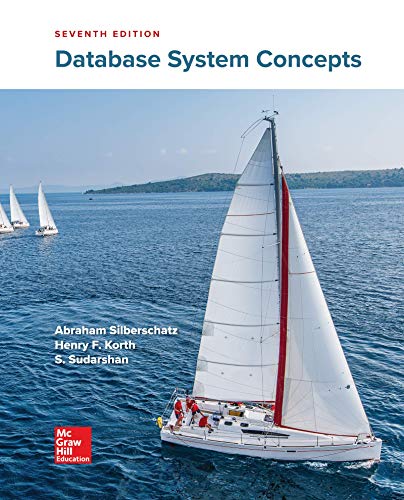
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
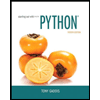
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
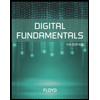
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
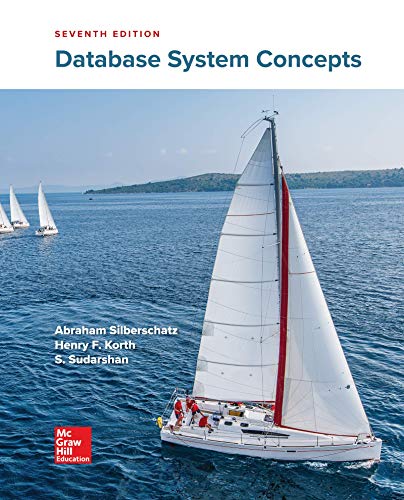
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
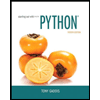
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
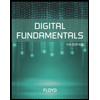
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
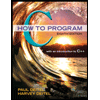
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
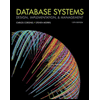
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
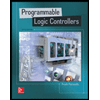
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education