Write a Program in C++ programming language to take three ints, a b c, print if it is possible to add two of the ints to get the third.
Write a Program in C++ programming language to take three ints, a b c, print if it is possible to add two of the ints to get the third.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:**C++ Programming Task:**
Write a program in the C++ programming language to take three integers, `a`, `b`, and `c`. The program should print a message indicating if it is possible to add any two of the integers to obtain the third integer.
---
**Overview:**
This task involves basic conditional checking in C++. The goal is to determine if any two numbers out of three given integers can be added together to equal the third integer. This is a fundamental exercise in understanding basic logic operations and conditional statements in programming.
---
**Example Solution:**
Here is an example of how you could accomplish this task in C++:
```cpp
#include <iostream>
using namespace std;
int main() {
int a, b, c;
cout << "Enter three integers: ";
cin >> a >> b >> c;
if (a + b == c || b + c == a || c + a == b) {
cout << "Yes, it is possible to add two of the integers to get the third." << endl;
} else {
cout << "No, it is not possible to add two of the integers to get the third." << endl;
}
return 0;
}
```
**Explanation:**
1. The program starts by including the necessary header file, `<iostream>`, to use input and output functions.
2. The `main()` function is defined, which will execute the core logic.
3. Three integers, `a`, `b`, and `c`, are declared.
4. The program prompts the user to enter three integers.
5. It reads the input integers using `cin`.
6. An `if` statement checks if the sum of any two integers equals the third integer.
7. The result of the check is printed with `cout`.
By following this example, users can understand how conditional checks work in C++ and how to read and process user inputs.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
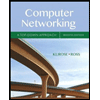
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
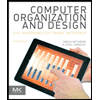
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
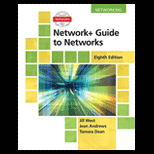
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
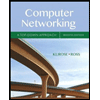
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
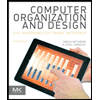
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
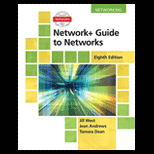
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
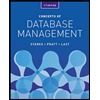
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
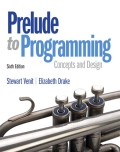
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
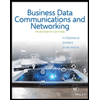
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY