Write a java program to determine the minimum operations required for list S to be transformed to list T Two operations to consider: shift(l) returns a new list after moving the front number to the back—i.e., it returns l[1:] + l[0] (in Python’s list notation.) reverse(l) returns the reverse of the l—i.e., it returns l[::-1] (in Python’s list notation.) Given a list S, come up with a list T such that T can be obtained from S by applying zero or more of shift and reverse operations in some order. As an example, consider S = {1, 2, 3, 4} and T = {2, 1, 4, 3}, we can see that T = shift(shift(reverse(S))) But this is not the only way to transform S to T. To illustrate, here are some other sequence of operations: T = reverse(shift(shift(S))) T = shift(reverse(shift(S))) T = reverse(shift(shift(reverse(reverse(S)))))) Our goal in this problem is to find the smallest number of operations to achieve this transformation. Some more input/ output examples of length 5 minimumOps(List.of(1,2,3,4,5), List.of(2,1,5,4,3)) == 3 minimumOps(List.of(5,4,3,2,1), List.of(1,5,4,3,2)) == 3 minimumOps(List.of(1,2,3,4,5), List.of(5,4,3,2,1)) == 1
Write a java program to determine the minimum operations required for list S to be transformed to list T
Two operations to consider:
-
shift(l) returns a new list after moving the front number to the back—i.e., it returns l[1:] + l[0] (in Python’s list notation.)
-
reverse(l) returns the reverse of the l—i.e., it returns l[::-1] (in Python’s list notation.)
-
Given a list S, come up with a list T such that T can be obtained from S by applying zero or more of shift and reverse operations in some order. As an example,
-
consider S = {1, 2, 3, 4} and T = {2, 1, 4, 3}, we can see that
T = shift(shift(reverse(S)))
But this is not the only way to transform S to T.
To illustrate, here are some other sequence of operations:
T = reverse(shift(shift(S)))
-
T = shift(reverse(shift(S)))
-
T = reverse(shift(shift(reverse(reverse(S))))))
Our goal in this problem is to find the smallest number of operations to achieve this transformation.
Some more input/ output examples of length 5
- minimumOps(List.of(1,2,3,4,5), List.of(2,1,5,4,3)) == 3 minimumOps(List.of(5,4,3,2,1), List.of(1,5,4,3,2)) == 3 minimumOps(List.of(1,2,3,4,5), List.of(5,4,3,2,1)) == 1

Step by step
Solved in 2 steps with 1 images

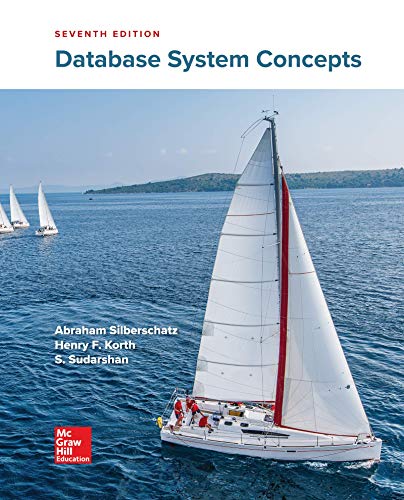
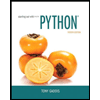
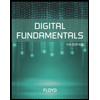
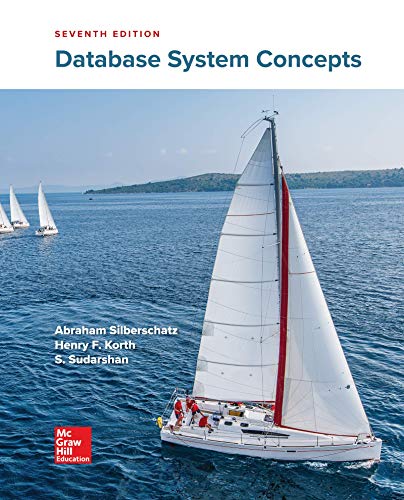
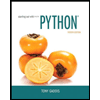
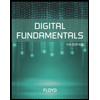
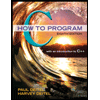
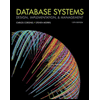
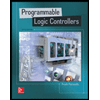