Write a JAVA program that reads a number between 1,000 and 999,999 from the user, where the user enters a comma in the input. Then print the number without a comma. Here is a sample dialog: Please enter an integer between 1,000 and 999,999: 24,562 24562 Hint: Read the input as a string. Measure the length of the string. Suppose it contains n characters. Then extract the substring consisting of the first n-4 characters and the last three characters
Write a JAVA program that reads a number between 1,000 and 999,999 from the user, where the user enters a comma in the input. Then print the number without a comma. Here is a sample dialog:
Please enter an integer between 1,000 and 999,999: 24,562
24562
Hint: Read the input as a string. Measure the length of the string. Suppose it contains n characters. Then extract the substring consisting of the first n-4 characters and the last three characters

Approach
Here we will first read the input as a string with comma and then we will print out subtring consisting of first n-4 characters (index 0 to length-5) and the last three characters (index length-3 to length-1).
Syntax: string.substring(int startingIndex, int lastIndex);
Here lastIndex is exclusive of the sustring.
Code
import java.util.*;
public class Main
{
public static void main(String[] args) {
Scanner sc= new Scanner(System.in);
System.out.print("Please enter an integer between 1,000 and 999,999: ");
String str= sc.nextLine();
int len = str.length();
if(len<5 || len >7 || str.charAt(len-4)!=','){
System.out.println("Input number entered is not correct");
}
System.out.println(str.substring(0,len-4)+str.substring(len-3,len));
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

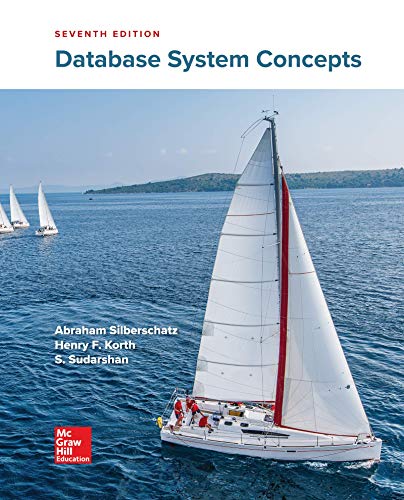
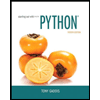
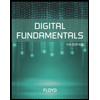
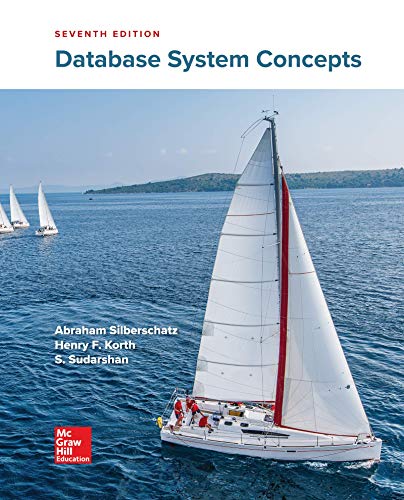
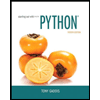
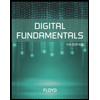
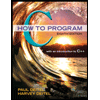
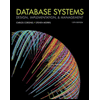
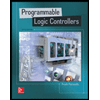