Write a Java program called Process marks (using files). The program must read the marks of students from a text file and process the marks. The results must be saved in a text file called result. 2.1 Write a Student class with the following instance fields: Name of student arrMarks (integer) // 4 marks out of 100 The class must contain a parameterised constructor and get methods for the two instance fields. Write a method called calcAve() to calculate and return the average mark. Write a method called didPass() to determine whether the student passes the module with an average of at least 50%. Return the word Pass or Fail. Write a toString() method to construct and return the following string of student data: Name of student 4 marks Peter Sampson 40 50 40 30 2.2 Create a text file called marks. Save the text file in the project folder. Example of the content: Peter Sampson#40#50#40#30 Diane Wilson#78#89#66#55 James Dube#87#98#78#88 2.3 Create a class called FileMethods. Write a readFile method to read the data from the marks text file into an array of Student objects. Write a writeFile method to write the name of the student, the student's average mark, and whether they pass the module or not to the text file called result. Example of text file content: Peter Samson 40 Fail Write get methods (accessors) for the array of objects and the counter variable. 2.4 Write a test class to call the methods. Write a static displayData() method to display the content of the array. Example of output: List of students Name Marks Ave Result Peter Sampson 40 50 40 30 40 Fail
Write a Java program called Process marks (using files). The program must read the marks of students from a text file and process the marks. The results must be saved in a text file called result.
2.1 Write a Student class with the following instance fields:
- Name of student
- arrMarks (integer) // 4 marks out of 100
The class must contain a parameterised constructor and get methods for the two instance fields.
Write a method called calcAve() to calculate and return the average mark.
Write a method called didPass() to determine whether the student passes the module with an average of at least 50%. Return the word Pass or Fail.
Write a toString() method to construct and return the following string of student data:
Name of student 4 marks
Peter Sampson 40 50 40 30
2.2 Create a text file called marks. Save the text file in the project folder.
- Example of the content:
Peter Sampson#40#50#40#30
Diane Wilson#78#89#66#55
James Dube#87#98#78#88
2.3 Create a class called FileMethods.
- Write a readFile method to read the data from the marks text file into an array of Student objects.
- Write a writeFile method to write the name of the student, the student's average mark, and whether they pass the module or not to the text file called result.
- Example of text file content:
Peter Samson 40 Fail
- Write get methods (accessors) for the array of objects and the counter variable.
2.4 Write a test class to call the methods. Write a static displayData() method to display the content of the array.
- Example of output:
List of students
Name Marks Ave Result
Peter Sampson 40 50 40 30 40 Fail

Step by step
Solved in 3 steps with 2 images

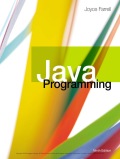
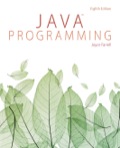
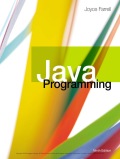
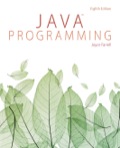