Write a java code for the following. Design a class named Person and its two subclasses named Student and Employee. Make Faculty and Staff subclasses of Employee. A person has a name, address, phone number, and email address. A student has a class status (freshman, sophomore, junior, or senior). Define the status as a constant. An employee has an office, salary, and date hired. Use the MyDate class defined as below to create an object for date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString method in each class to display the class name and the person’s name. Write a test program that creates a Person, Student, Employee, Faculty, and Staff, and invokes their toString() methods. Design a class named MyDate. The class contains: The data fields year, month, and day that represent a date. month is 0-based, i.e., 0 is for January. A no-arg constructor that creates a MyDate object for the today’s date. A constructor that constructs a MyDate object with a specified elapsed time since midnight, January 1, 1970, in milliseconds. A constructor that constructs a MyDate object with the specified year, month, and day. Three getter methods for the data fields year, month, and day, respectively. A method named setDate(long elapsedTime) that sets a new date for the object using the elapsed time.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Write a java code for the following.
Design a class named Person and its two subclasses named Student and Employee. Make Faculty and Staff subclasses of Employee. A person has a name, address, phone number, and email address. A student has a class status (freshman, sophomore, junior, or senior). Define the status as a constant. An employee has an office, salary, and date hired. Use the MyDate class defined as below to create an object for date hired. A faculty member has office hours and a rank. A staff member has a title. Override the toString method in each class to display the class name and the person’s name. Write a test program that creates a Person, Student, Employee, Faculty, and Staff, and invokes their toString() methods.
Design a class named MyDate. The class contains:
The data fields year, month, and day that represent a date. month is 0-based, i.e., 0 is for January.
A no-arg constructor that creates a MyDate object for the today’s date.
A constructor that constructs a MyDate object with a specified elapsed time since midnight, January 1, 1970, in milliseconds.
A constructor that constructs a MyDate object with the specified year, month, and day.
Three getter methods for the data fields year, month, and day, respectively.
A method named setDate(long elapsedTime) that sets a new date for the object using the elapsed time.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

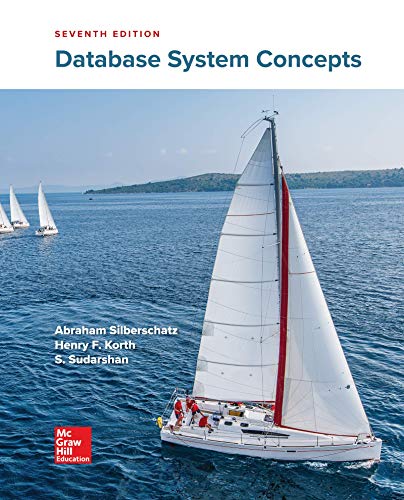
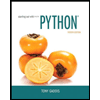
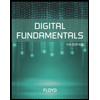
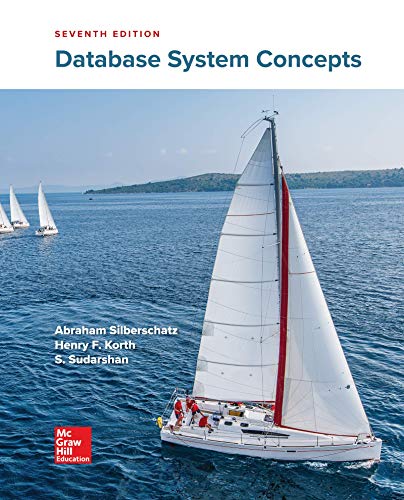
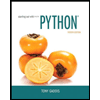
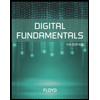
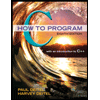
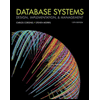
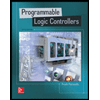