Write a Java class called GuessMyNumber that prompts the user for an integer n, tells the user to think of a number between 0 and n−1, then makes guesses as to what the number is. After each guess, the program must ask the user if the number is lower, higher, or correct. You must implement the divide-and-conquer algorithm from class. In particu[1]lar, you should round up when the middle of your range is in between two integers. (For example, if your range is 0 to 31, you should guess 16 and not 15, but if your range is 0 to 30 you should certainly guess 15). The flow should look like the following: Enter n: 32 Welcome to Guess My Number! Please think of a number between 0 and 31. Is your number: 16? Please enter C for correct, H for too high, or L for too low. Enter your response (H/L/C): H Is your number: 8? Please enter C for correct, H for too high, or L for too low. Enter your response (H/L/C): L Is your number: 12? Please enter C for correct, H for too high, or L for too low. Enter your response (H/L/C): C Thank you for playing Guess My Number! As part of your implementation, you should check that n is not 0 or negative. (You need not worry about the case where the user enters a non-integer). You should also check that the user is entering one of the letters H, L, or C each time your program makes a guess. This flow should look like the following: Enter n: -1 Enter a positive integer for n: 32 Welcome to Guess My Number! Please think of a number between 0 and 31. Is your number: 16? Please enter C for correct, H for too high, or L for too low. Enter your response (H/L/C): asdf Enter your response (H/L/C): H Is your number: 8? ...
Write a Java class called GuessMyNumber that prompts the
user for an integer n, tells the user to think of a number between 0 and
n−1, then makes guesses as to what the number is. After each guess, the
program must ask the user if the number is lower, higher, or correct. You
must implement the divide-and-conquer
integers. (For example, if your range is 0 to 31, you should guess 16 and
not 15, but if your range is 0 to 30 you should certainly guess 15). The
flow should look like the following:
Enter n: 32
Welcome to Guess My Number!
Please think of a number between 0 and 31.
Is your number: 16?
Please enter C for correct, H for too high, or L for too low.
Enter your response (H/L/C): H
Is your number: 8?
Please enter C for correct, H for too high, or L for too low.
Enter your response (H/L/C): L
Is your number: 12?
Please enter C for correct, H for too high, or L for too low.
Enter your response (H/L/C): C
Thank you for playing Guess My Number!
As part of your implementation, you should check that n is not 0 or
negative. (You need not worry about the case where the user enters a
non-integer). You should also check that the user is entering one of the
letters H, L, or C each time your program makes a guess. This flow should
look like the following:
Enter n: -1
Enter a positive integer for n: 32
Welcome to Guess My Number!
Please think of a number between 0 and 31.
Is your number: 16?
Please enter C for correct, H for too high, or L for too low.
Enter your response (H/L/C): asdf
Enter your response (H/L/C): H
Is your number: 8?
...

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

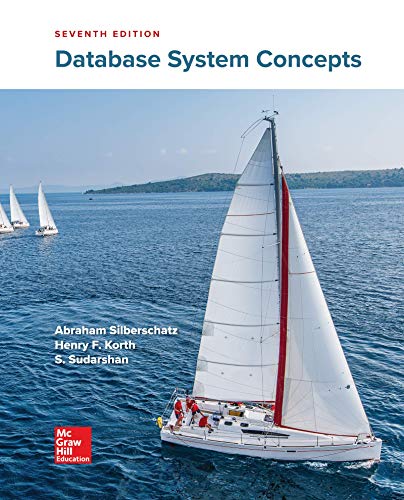
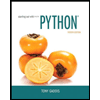
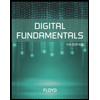
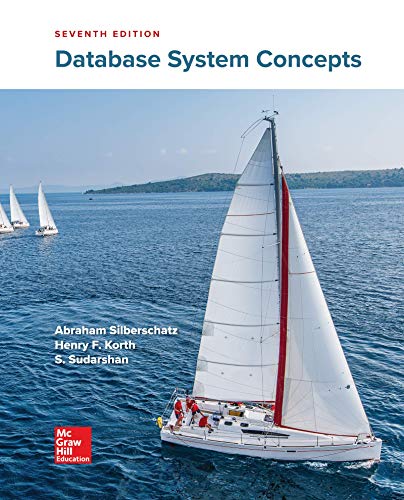
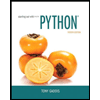
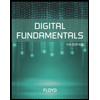
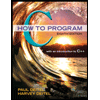
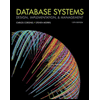
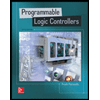