Write a function to check three given integers and return their sum. However, If one of the values is the same as another of the values, then both the values are not counted in the sum. In Ruby language.


#function to check three integers and return their sum
def check_sum(num1, num2, num3)
#Check numbers
if num1==num2 && num2==num3
#if all numbers are same return 0 as sum
return 0
elsif num1==num2
#if num1 and num2 is same return num3 as sum
return num3
elsif num1==num3
#if num1 and num3 is same return num2 as sum
return num2
elsif num2==num3
#if num2 and num3 is same return num1 as sum
return num1
else
#if all number is different return their sum
return num1+num2+num3
end
end
#test code
print "Sum(6,6,6): ",check_sum(6, 6, 6),"\n"
print "Sum(6,6,4): ",check_sum(6, 6, 4),"\n"
print "Sum(6,3,6): ",check_sum(6, 3, 6),"\n"
print "Sum(2,6,6): ",check_sum(2, 6, 6),"\n"
print "Sum(2,3,4): ",check_sum(2, 3, 4),"\n"
Step by step
Solved in 2 steps

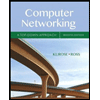
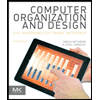
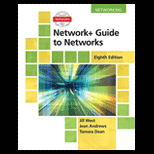
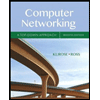
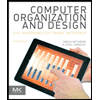
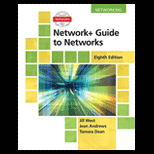
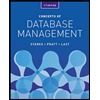
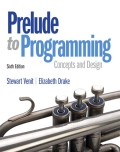
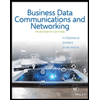