Write a function that hides the characters in a string, yielding a string of equal length where each character is replaced by a *. For example, the call hide_characters("secret") should return the string strings.cpp 1#include z using namespace std; :/** Returns a string of asterisks of the same length as a given string. @param str a string such as "secret" @return a string with each character of str changed to a *, such as "******* 4 / *5 ]string hide_characters(string str) 1{ int n = str.length(); for(int i=0 ; i < n; i++) { string s ; S = s + "*"; return s ; }
Write a function that hides the characters in a string, yielding a string of equal length where each character is replaced by a *. For example, the call hide_characters("secret") should return the string strings.cpp 1#include z using namespace std; :/** Returns a string of asterisks of the same length as a given string. @param str a string such as "secret" @return a string with each character of str changed to a *, such as "******* 4 / *5 ]string hide_characters(string str) 1{ int n = str.length(); for(int i=0 ; i < n; i++) { string s ; S = s + "*"; return s ; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Why those two codes were not math?
I'm really confused, I think those two codes should be the same, but the first one is not working, could you give me some advice? or better code idea.
thanks

Transcribed Image Text:# Educational Content: Hiding Characters in a String
## Introduction
In this tutorial, we learn how to write a C++ function that takes a string as input and returns a new string of the same length with each character replaced by an asterisk (*). This function is useful for concealing sensitive information.
## Example
For the input string "secret", the function will return "******".
## Source Code
```cpp
#include <string>
using namespace std;
/**
* Returns a string of asterisks of the same length as a given string.
* @param str a string such as "secret"
* @return a string with each character of str changed to a *, such as "******".
*/
string hide_characters(string str)
{
int n = str.length();
string s;
for(int i = 0; i < n; i++)
{
s = s + "*";
}
return s;
}
```
## Explanation
- **Include Directives**:
- `#include <string>`: This includes the string library to handle string objects in C++.
- **Namespace**:
- `using namespace std;` allows access to standard C++ library features without prefixing them with `std::`.
- **Function**:
- `string hide_characters(string str)`:
- **Parameters**: Takes a single parameter `str` of type `string`.
- **Returns**: A string of asterisks with the same length as the input string.
- **Logic**:
- Determine the length of the input string using `str.length()`.
- Initialize an empty string `s`.
- Use a for loop to append an asterisk to `s` for each character of `str`.
- Return the resultant string `s`.
This function efficiently conceals the content of the input string by replacing each character with an asterisk, maintaining the original string's length.

Transcribed Image Text:```cpp
#include <string>
using namespace std;
/**
Returns a string of asterisks of the same length as
a given string.
@param str a string such as "secret"
@return a string with each character of str changed to a *,
such as "******".
*/
string hide_characters(string str)
{
string s;
for(int i = 0; i < str.length(); i++) s = s + "*";
return s;
}
```
### Explanation
The code is from a C++ program file named `strings.cpp`. This program includes a function called `hide_characters` which takes a string as a parameter and returns a new string of the same length, but with each character replaced by an asterisk (*).
- **Libraries Included:**
- `<string>`: This library is included to use string operations in C++.
- **Namespace Used:**
- `std`: The standard namespace is used, which allows the use of standard C++ functions and objects without needing to prepend `std::` before them.
- **Function Explanation:**
- `string hide_characters(string str)`: This function accepts a single parameter, `str`, which is a string. It initializes an empty string `s` and uses a `for` loop that iterates over each character in `str`, appending an asterisk to `s` for each iteration. Finally, it returns the string `s`, which contains asterisks equivalent to the length of the input string `str`.
**Code Execution:**
- **CodeCheck and Reset**: The interface suggests options like "CodeCheck" to probably validate the code and "Reset" to revert any changes made to the code during editing.
This program could be used to mask sensitive information, like passwords or secrets, when displaying them in environments where the text visibility should be restricted.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
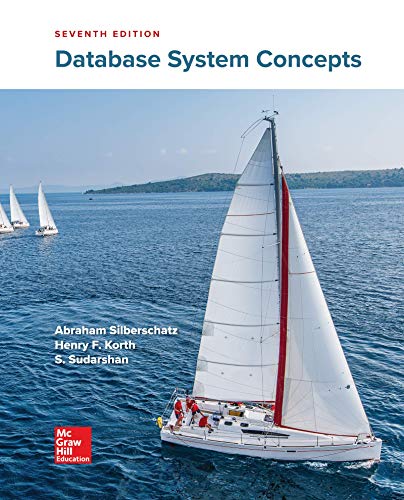
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
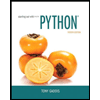
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
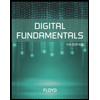
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
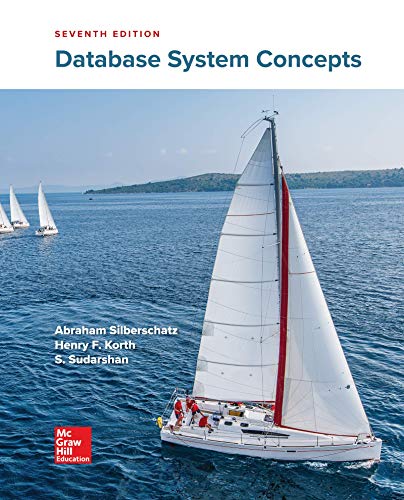
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
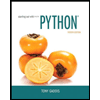
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
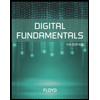
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
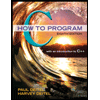
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
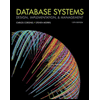
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
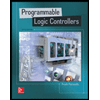
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education