"Write a function that gets a string, as presented below, as a parameter and returns the user name and the host from the string.\n", "\n", "'From stephen.marquard@uct.ac.za Sat Jan 5 09:14:16 2008'\n", #t
"Write a function that gets a string, as presented below, as a parameter and returns the user name and the host from the string.\n", "\n", "'From stephen.marquard@uct.ac.za Sat Jan 5 09:14:16 2008'\n", #t
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
python

Transcribed Image Text:### Extracting User and Host from an Email String
**Problem Statement:**
Write a function that receives a string, as presented below, as a parameter and returns the username and the host from the string.
**Example String:**
```
'From stephen.marquard@uct.ac.za Sat Jan 5 09:14:16 2008'
```
**Detailed Breakdown:**
1. **Original String:**
```
'From stephen.marquard@uct.ac.za Sat Jan 5 09:14:16 2008'
```
2. **Extracted Information:**
- **User:**
```
stephen.marquard
```
- **Host:**
```
uct.ac.za
```
Given the string in the example, the function should correctly identify and separate the username (`stephen.marquard`) and the host (`uct.ac.za`) parts of the email address.
This type of function is useful in various applications such as email processing, user identity management, and data parsing tasks in software development.
**Python Implementation:**
Here’s an example of how you might implement such a function in Python:
```python
def extract_user_host(email_string):
import re
pattern = r'From (\S+@\S+)'
match = re.search(pattern, email_string)
if match:
email = match.group(1)
user, host = email.split('@')
return user, host
return None
# Example usage
email_string = 'From stephen.marquard@uct.ac.za Sat Jan 5 09:14:16 2008'
user, host = extract_user_host(email_string)
print("The user is:", user)
print("The host is:", host)
```
**Output:**
```
The user is: stephen.marquard
The host is: uct.ac.za
```
This implementation uses a regular expression to find the email within the string and then splits the email to obtain the user and host parts.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
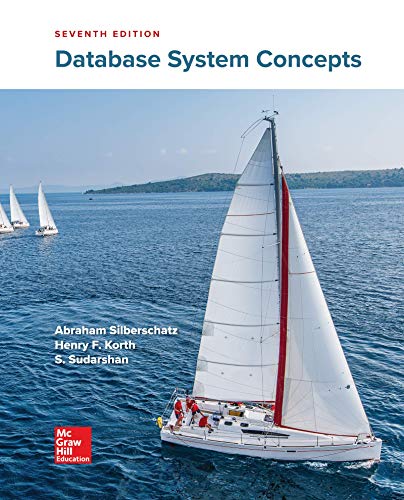
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
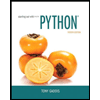
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
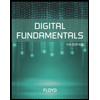
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
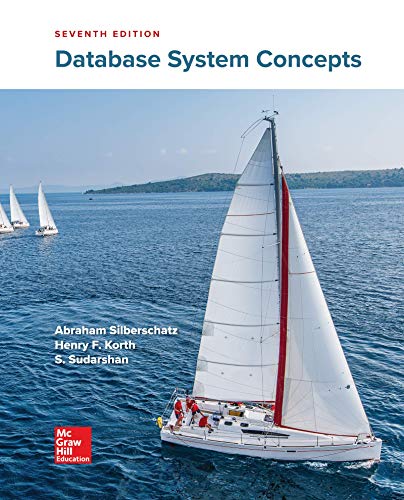
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
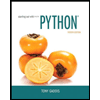
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
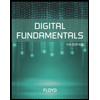
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
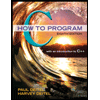
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
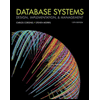
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
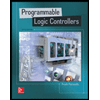
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education