Write a function named all-unique in Scheme that takes a list as input and returns true if each item appears once only on the list and false otherwise. You may assume the list is flat (i.e., no nested lists). Hint: Consider writing a helper function contains that returns wither a list contains a given item. For example, (all-unique '(a b c 1 2)) should return true • (all-unique '(a b c 1 a)) should return false • (all-unique '()) should return true
Write a function named all-unique in Scheme that takes a list as input and returns true if each item appears once only on the list and false otherwise. You may assume the list is flat (i.e., no nested lists). Hint: Consider writing a helper function contains that returns wither a list contains a given item. For example, (all-unique '(a b c 1 2)) should return true • (all-unique '(a b c 1 a)) should return false • (all-unique '()) should return true
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
in Scheme please, i need help

Transcribed Image Text:### Scheme Programming: Implementing an `all-unique` Function
This educational page demonstrates how to write a Scheme function named `all-unique`. The goal of the function is to examine a provided list and return `true` if every element appears only once. Conversely, if any elements are duplicated, the function should return `false`. The list is assumed to be flat, without nested lists.
#### Instructions:
1. **Function Specifications:**
- **Function Name:** `all-unique`
- **Input:** A single list.
- **Output:** A boolean value (`true` or `false`).
2. **Implementation Considerations:**
- Consider creating a helper function, `contains`, that checks whether a given item exists within a list.
3. **Example Usage:**
- `(all-unique '(a b c 1 2))` should return `true`.
- `(all-unique '(a b c 1 a))` should return `false`.
- `(all-unique '())` should return `true`.
#### Code Example:
Below is a sample code snippet showing a partial implementation of the `all-unique` function in Scheme.
```scheme
(define (all-unique lst def)
(if (and (null? def)
(if (equal? lst) 0))
'()
)
(if all-unique lst ((!- (all-unique lst ))
```
**Note:** The code shown above is incomplete and serves as an illustrative starting point for building the `all-unique` function. It is important to further develop the logic to handle list iteration and uniqueness checks effectively.
Expert Solution

Step 1
Python Programming language :
Python is a well-liked general-purpose programming language that has a large toolkit for use in industries like data research and web development. It is also noted for being simple to read. Similar to other high-level languages like Java and JavaScript, the programming language supports a variety of paradigms including functional, procedural, and object-oriented programming (oop).
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
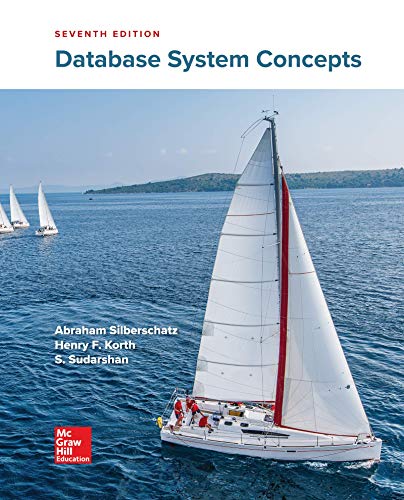
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
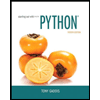
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
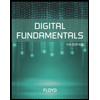
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
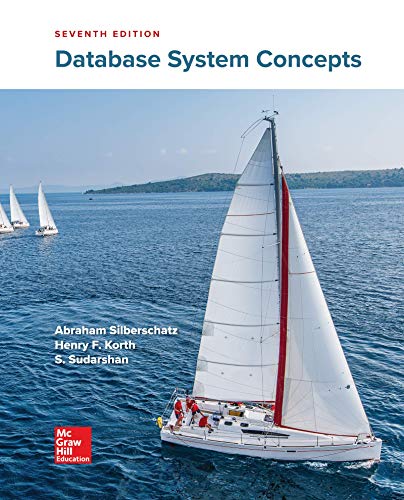
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
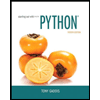
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
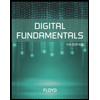
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
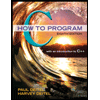
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
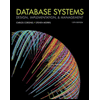
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
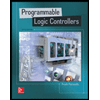
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education