Write a function in python, build_list(), to read words from standard input and store them in a list. You may assume that there will be fewer than 100 words. If the string "STOP" is given as input before 100 words have been added to the list, stop the for loop and return the accumulated list of strings. Hints: - Use the accumulate-a-list pattern, with acc_list initialized to the empty list, [] - Use a for loop to iterate i over range(100) - When STOP is read from input, break from the for loop; otherwise, append the new word to acc_list before reading the next input - Return acc_list For example: Test Input Result L = build_list() print(L) C = ["April", "showers", "bring", "May", "flowers"] if L != C: print("error") April showers bring May flowers STOP ['April', 'showers', 'bring', 'May', 'flowers']
Write a function in python, build_list(), to read words from standard input and store them in a list.
- You may assume that there will be fewer than 100 words.
- If the string "STOP" is given as input before 100 words have been added to the list, stop the for loop and return the accumulated list of strings.
Hints:
- Use the accumulate-a-list pattern, with acc_list initialized to the empty list, []
- Use a for loop to iterate i over range(100)
- When STOP is read from input, break from the for loop; otherwise, append the new word to acc_list before reading the next input
- Return acc_list
For example:
Test | Input | Result |
---|---|---|
L = build_list() print(L) C = ["April", "showers", "bring", "May", "flowers"] if L != C: print("error") | April showers bring May flowers STOP | ['April', 'showers', 'bring', 'May', 'flowers'] |

Here is the approach :
1) Create the method called as build_list() first
2) Inside the function create the list called as acc_list
3) create for loop in the range of 0 to 100
4) Take the input in the for loop
5) Check if the input word is stop or not . If yes then stop the loop and print the list to the console .
6)If no then add the number in the list
Step by step
Solved in 2 steps with 2 images

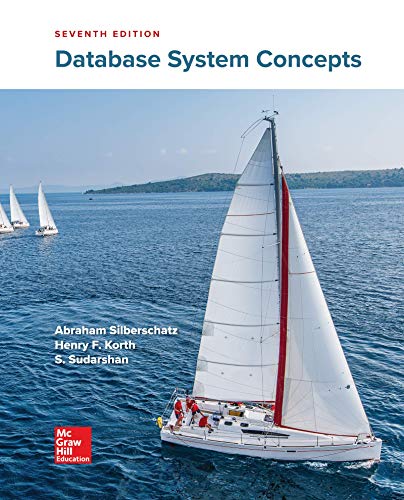
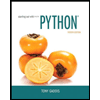
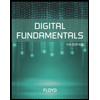
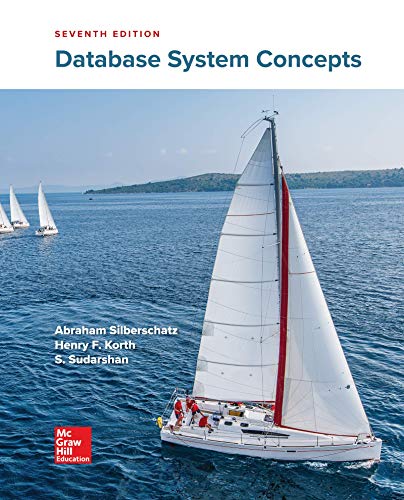
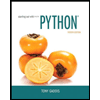
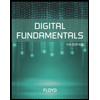
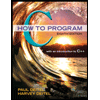
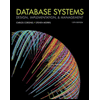
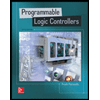