Write a function DrivingCost with input parameters drivenMiles, milesPerGallon, and dollarsPerGallon,
Write a function DrivingCost with input parameters drivenMiles, milesPerGallon, and dollarsPerGallon,
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:**Driving Cost Calculation Using Functions**
In this activity, you will write a function called `DrivingCost` that will help calculate the dollar cost of driving a certain number of miles. The function will take three input parameters:
- `drivenMiles`: the number of miles to drive.
- `milesPerGallon`: the vehicle's fuel efficiency in miles per gallon.
- `dollarsPerGallon`: the cost of gas per gallon.
The goal is to determine the cost in dollars to drive the specified number of miles. All inputs are of type `double`. For instance, if the function is called with the values `(50, 20.0, 3.1599)`, it should return `7.89975`.
### Task:
Define the `DrivingCost` function within a program that:
- Takes the car's efficiency in `miles/gallon` and the gas cost in `dollars/gallon` as inputs.
- Calculates the gas cost for three specific mile values: 10 miles, 50 miles, and 400 miles.
- Outputs the resulting costs formatted to two decimal places. You can use the following code to format the output:
```c
printf("%.2lf", yourValue);
```
**Example Input:**
```
20.0 3.1599
```
**Example Output:**
```
1.58 7.90 63.20
```
### Function Definition:
Your program must define and call the function:
```c
double DrivingCost(double drivenMiles, double milesPerGallon, double dollarsPerGallon)
```
### Template:
Below is the template for your program. Fill in the necessary code to complete the function and call it appropriately.
```c
#include <stdio.h>
/* Define your function here */
int main(void) {
/* Type your code here. */
return 0;
}
```
**Note:** You will need to implement the logic to compute the driving cost in the `DrivingCost` function and ensure it integrates with the main program logic to produce the expected output.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Recommended textbooks for you
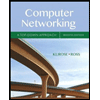
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
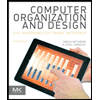
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
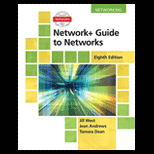
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
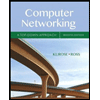
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
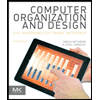
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
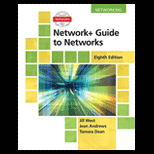
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
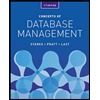
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
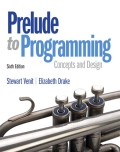
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
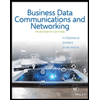
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY