Write a few words about why we've created each of the tests above. What do they cover? Why do we need them? ===================================================================================== @Test @Order(4) publicvoidverifyEmailIntegrationTest()throwsException{ /* Check if the User exists */ Optionalopt=this.userRepository.findByUsername(this.user.getUsername()); assertTrue(opt.isPresent(),"User Should Exist"); /* Check the user emailVerified flag initial value */ assertEquals(false,opt.get().getEmailVerified()); Stringjwt=String.format("Bearer %s",this.jwtService.generateJwtToken(this.user.getUsername(),10_000)); /* Check the Rest End Point Response */ this.mockMvc.perform(MockMvcRequestBuilders.get("/user/verify/email").header(AUTHORIZATION,jwt)).andExpect(status().isOk()); /* Check if the User exists */ opt=this.userRepository.findByUsername(this.user.getUsername()); assertTrue(opt.isPresent(),"User Should Exist"); /* Check the user emailVerified flag was updated */ assertEquals(true,opt.get().getEmailVerified()); } @Test @Order(5) publicvoidverifyEmailUsernameNotFoundIntegrationTest()throwsException{ /* Check if the User exists */ Optionalopt=this.userRepository.findByUsername(this.otherUsername); assertTrue(opt.isEmpty(),"User Should Not Exist"); Stringjwt=String.format("Bearer %s",this.jwtService.generateJwtToken(this.otherUsername,10_000)); /* Check the Rest End Point Response */this.mockMvc.perform(MockMvcRequestBuilders.get("/user/verify/email").header(AUTHORIZATION,jwt)) .andExpect(status().is4xxClientError()).andExpect(jsonPath("$.httpStatusCode",is(400))) .andExpect(jsonPath("$.httpStatus",is("BAD_REQUEST"))) .andExpect(jsonPath("$.reason",is("BAD REQUEST"))) .andExpect(jsonPath("$.message",is(String.format("Username doesn't exist, %s",this.otherUsername)))); } @Test @Order(6) publicvoidloginIntegrationTest()throwsException{ /* Create object to generate JSON */ ObjectNoderoot=this.objectMapper.createObjectNode(); root.put("username",this.user.getUsername()); root.put("password",this.user.getPassword()); /* Check the Rest End Point Response */ this.mockMvc.perform(MockMvcRequestBuilders.post("/user/login") .contentType(MediaType.APPLICATION_JSON) .content(this.objectMapper.writeValueAsString(root))) .andExpect(header().exists(AUTHORIZATION)) .andExpect(status().isOk()) .andExpect(jsonPath("$.firstName",is(this.user.getFirstName()))) .andExpect(jsonPath("$.lastName",is(this.user.getLastName()))) .andExpect(jsonPath("$.username",is(this.user.getUsername()))) .andExpect(jsonPath("$.phone",is(this.user.getPhone()))) .andExpect(jsonPath("$.emailId",is(this.user.getEmailId()))) .andExpect(jsonPath("$.emailVerified",is(true))); } @Test @Order(7) publicvoidloginEmailNotVerifiedIntegrationTest()throwsException{ /* Create object to generate JSON */ ObjectNoderoot=this.objectMapper.createObjectNode(); root.put("username",this.user.getUsername()); root.put("password",this.user.getPassword()); /* Check if the User exists */ Optionalopt=this.userRepository.findByUsername(this.user.getUsername()); assertTrue(opt.isPresent(),"User Should Exist"); /* Set user.emailVerified to false */ opt.ifPresent(u->{u.setEmailVerified(false); this.userRepository.save(u);}); /* Check the Rest End Point Response */ this.mockMvc.perform(MockMvcRequestBuilders.post("/user/login") .contentType(MediaType.APPLICATION_JSON) .content(this.objectMapper.writeValueAsString(root))) .andExpect(status().is4xxClientError()) .andExpect(jsonPath("$.httpStatusCode",is(400))) .andExpect(jsonPath("$.httpStatus",is("BAD_REQUEST"))) .andExpect(jsonPath("$.reason",is("BAD REQUEST"))) .andExpect(jsonPath("$.message",is(String.format("Email requires verification, %s",this.user.getEmailId())))); /* Check if the User exists */ opt=this.userRepository.findByUsername(this.user.getUsername()); assertTrue(opt.isPresent(),"User Should Exist");
Write a few words about why we've created each of the tests above. What do they cover? Why do we need them?
=====================================================================================
@Test
@Order(4)
publicvoidverifyEmailIntegrationTest()throwsException{
/* Check if the User exists */
Optional<User>opt=this.userRepository.findByUsername(this.user.getUsername());
assertTrue(opt.isPresent(),"User Should Exist");
/* Check the user emailVerified flag initial value */
assertEquals(false,opt.get().getEmailVerified());
Stringjwt=String.format("Bearer %s",this.jwtService.generateJwtToken(this.user.getUsername(),10_000));
/* Check the Rest End Point Response */
this.mockMvc.perform(MockMvcRequestBuilders.get("/user/verify/email").header(AUTHORIZATION,jwt)).andExpect(status().isOk());
/* Check if the User exists */
opt=this.userRepository.findByUsername(this.user.getUsername());
assertTrue(opt.isPresent(),"User Should Exist");
/* Check the user emailVerified flag was updated */
assertEquals(true,opt.get().getEmailVerified());
}
@Test
@Order(5)
publicvoidverifyEmailUsernameNotFoundIntegrationTest()throwsException{
/* Check if the User exists */
Optional<User>opt=this.userRepository.findByUsername(this.otherUsername);
assertTrue(opt.isEmpty(),"User Should Not Exist");
Stringjwt=String.format("Bearer %s",this.jwtService.generateJwtToken(this.otherUsername,10_000));
/* Check the Rest End Point Response */this.mockMvc.perform(MockMvcRequestBuilders.get("/user/verify/email").header(AUTHORIZATION,jwt))
.andExpect(status().is4xxClientError()).andExpect(jsonPath("$.httpStatusCode",is(400)))
.andExpect(jsonPath("$.httpStatus",is("BAD_REQUEST")))
.andExpect(jsonPath("$.reason",is("BAD REQUEST")))
.andExpect(jsonPath("$.message",is(String.format("Username doesn't exist, %s",this.otherUsername))));
}
@Test
@Order(6)
publicvoidloginIntegrationTest()throwsException{
/* Create object to generate JSON */
ObjectNoderoot=this.objectMapper.createObjectNode();
root.put("username",this.user.getUsername());
root.put("password",this.user.getPassword());
/* Check the Rest End Point Response */
this.mockMvc.perform(MockMvcRequestBuilders.post("/user/login")
.contentType(MediaType.APPLICATION_JSON)
.content(this.objectMapper.writeValueAsString(root)))
.andExpect(header().exists(AUTHORIZATION))
.andExpect(status().isOk())
.andExpect(jsonPath("$.firstName",is(this.user.getFirstName())))
.andExpect(jsonPath("$.lastName",is(this.user.getLastName())))
.andExpect(jsonPath("$.username",is(this.user.getUsername())))
.andExpect(jsonPath("$.phone",is(this.user.getPhone())))
.andExpect(jsonPath("$.emailId",is(this.user.getEmailId())))
.andExpect(jsonPath("$.emailVerified",is(true)));
}
@Test
@Order(7)
publicvoidloginEmailNotVerifiedIntegrationTest()throwsException{
/* Create object to generate JSON */
ObjectNoderoot=this.objectMapper.createObjectNode();
root.put("username",this.user.getUsername());
root.put("password",this.user.getPassword());
/* Check if the User exists */
Optional<User>opt=this.userRepository.findByUsername(this.user.getUsername());
assertTrue(opt.isPresent(),"User Should Exist");
/* Set user.emailVerified to false */
opt.ifPresent(u->{u.setEmailVerified(false);
this.userRepository.save(u);});
/* Check the Rest End Point Response */
this.mockMvc.perform(MockMvcRequestBuilders.post("/user/login")
.contentType(MediaType.APPLICATION_JSON)
.content(this.objectMapper.writeValueAsString(root)))
.andExpect(status().is4xxClientError())
.andExpect(jsonPath("$.httpStatusCode",is(400)))
.andExpect(jsonPath("$.httpStatus",is("BAD_REQUEST")))
.andExpect(jsonPath("$.reason",is("BAD REQUEST")))
.andExpect(jsonPath("$.message",is(String.format("Email requires verification, %s",this.user.getEmailId()))));
/* Check if the User exists */
opt=this.userRepository.findByUsername(this.user.getUsername());
assertTrue(opt.isPresent(),"User Should Exist");
}

Step by step
Solved in 4 steps

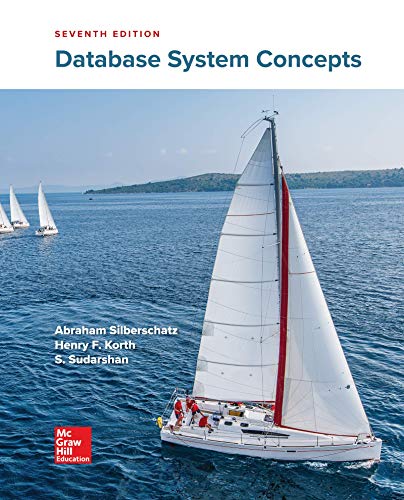
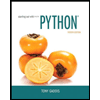
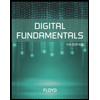
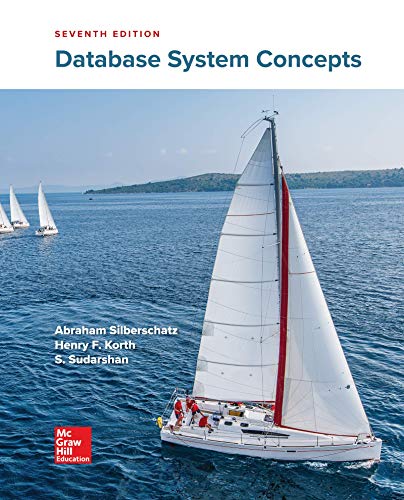
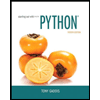
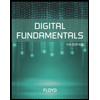
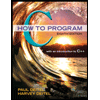
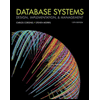
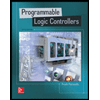