Write a C++ program using functions that will process a payroll for employees in a company based on the program specifications: Program specifications: The program should accept employee first name, number of hours worked, the hourly pay rate for each employee and calculate the state taxes, federal taxes, social security, medical cost, total deductions, gross pay and net pay for each employee. Implement the following functions: Write one function to get all inputs Write one function to calculate the gross pay Write one function to calculate state deductions Write one function to calculate federal deductions Write one function to calculate social security deductions Write one function to calculate medical deductions Write one function to calculate total deductions Write one function to calculate the net pay Write one function to display your output
Write a C++ program using functions that will process a payroll for employees in a company based on the program specifications:
Program specifications:
The program should accept employee first name, number of hours worked, the hourly pay rate for each employee and calculate the state taxes, federal taxes, social security, medical cost, total deductions, gross pay and net pay for each employee.
Implement the following functions:
- Write one function to get all inputs
- Write one function to calculate the gross pay
- Write one function to calculate state deductions
- Write one function to calculate federal deductions
- Write one function to calculate social security deductions
- Write one function to calculate medical deductions
- Write one function to calculate total deductions
- Write one function to calculate the net pay
- Write one function to display your output
Deductions:
State tax rate = .07
Federal tax rate = .15
Social Security = .17
Medical = .03
Note: all deductions should be declared as CONSTANTS
Note: NO Data Validations Required
Sample output:
Company Name
Employee Name Hours Worked Pay Rate Gross Pay Net Pay
--------------------- ------------------- ----------- ------------ ----------
John 38 15.50 X X.xx X X.xx
Deductions:
Deduction Type Deduction Rate Deduction Amount
-------------------- -------------------- -------------------------
State Taxes .07 X X.xx
Federal Taxes .15 X X.xx
Social Security .17 X X.xx
Medical .03 X X.xx
Grading Criteria:
Use of mnemonic variable names:....................................... 10
Use of constants..................................................................... 8
Input function...................................................................... 10
Gross pay function................................................................. 8
State deductions function....................................................... 8
Federal deductions function.................................................... 8
Social Security deductions function..................................... 8
Medical deductions function................................................... 8
Total deductions function....................................................... 8
Net pay function.................................................................... 8
Output function.................................................................... 16

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

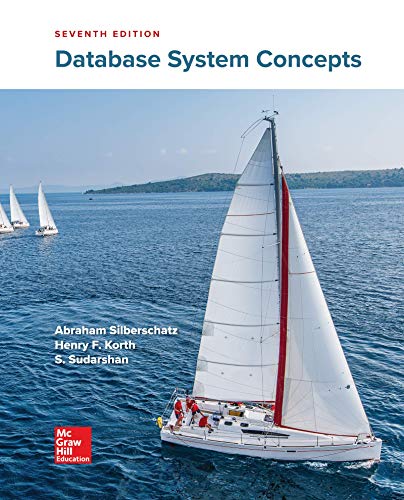
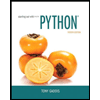
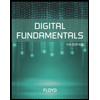
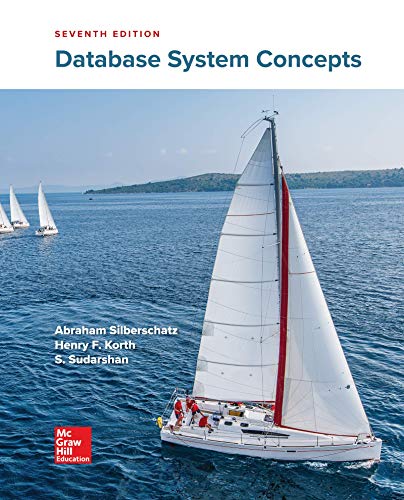
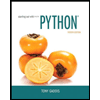
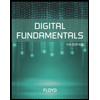
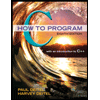
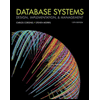
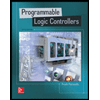