Write a C++ program to overload the increment and decrement operator.
USE TEMPLATE PROVIDED AT END WHEN MAKING SOLUTION.MATCH OUTPUT AS IT IS
-------------------------------------
Write a C++ program to overload the increment and decrement operator.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.
Consider a class named Contest and include the following private member variables.
Datatype |
Variable |
int |
number1 |
int |
number2 |
int |
number3 |
int |
number4 |
int |
number5 |
Create a parameterized constructor with arguments in order Event(int number1,int number2,int number3,int number4,int number5)
Include the following public methods in the class Contest.
Method Name |
Description |
void show(void) |
This function is used to display the five numbers. |
void operator ++() |
This function is used to add each number by one using the '++' operator. |
void operator --() |
This function is used to subtract each number by one using the '--' operator |
Input and Output Format:
Refer to sample input and output for formatting specifications.
[All text in bold corresponds to input and the rest corresponds to output]
Sample Input and Output 1 :
Enter the first number
9
Enter the second number
12
Enter the third number
20
Enter the fourth number
38
Enter the fifth number
19
Specify the option
plus
Response for plus
10, 13, 21, 39, 20
Specify the option
plus
Response for plus
11, 14, 22, 40, 21
Specify the option
plus
Response for plus
12, 15, 23, 41, 22
Specify the option
plus
Response for plus
13, 16, 24, 42, 23
Specify the option
minus
Response for minus
12, 15, 23, 41, 22
Specify the option
plus
Response for plus
13, 16, 24, 42, 23
Specify the option
minus
Response for minus
12, 15, 23, 41, 22
Specify the option
stop
------------------------TEMPLATES BELOW--------------------
MAIN.CPP
#include<cstring> #include<iostream> #include<string> #include "Contest.cpp" using namespace std; int main() { //Fill your code here } |
CONTEST.CPP
using namespace std; class Contest void operator ++() { void operator --() { }; |

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

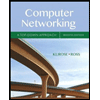
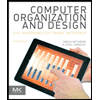
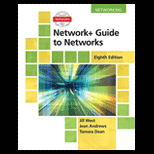
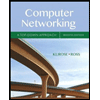
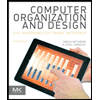
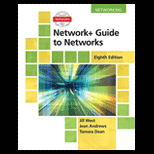
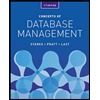
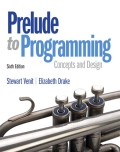
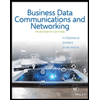