Write a C++ program to generate a random x in the range [1,9] and another random number y in the range [11,99]. Print the value of y/x in the output.
Write a C++ program to generate a random x in the range [1,9] and another random number y in the range [11,99]. Print the value of y/x in the output.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![**Title: Generating Random Numbers and Calculating Quotients using C++**
**Objective:**
Learn how to generate random numbers in C++ and perform simple arithmetic operations. This example focuses on generating two random numbers within specified ranges and finding their quotient.
**Task Description:**
Write a C++ program to generate a random integer `x` in the range [1, 9] and another random integer `y` in the range [11, 99]. The program should then calculate and print the value of the quotient `y/x`.
**Implementation Details:**
1. **Import Required Libraries:**
- Use `<iostream>` for input and output.
- Use `<cstdlib>` and `<ctime>` for generating random numbers.
2. **Generate Random Numbers:**
- Initialize the random seed using `srand(time(0))` to ensure different outputs each time the program runs.
- Generate `x` using `rand() % 9 + 1` to ensure `x` is between 1 and 9.
- Generate `y` using `rand() % 89 + 11` for a range between 11 and 99.
3. **Calculate and Print the Quotient:**
- Perform the division of the two random numbers (`y/x`).
- Print the result to the console.
**Sample Code:**
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
// Initialize random seed
srand(time(0));
// Generate random numbers
int x = rand() % 9 + 1; // Random number between 1 and 9
int y = rand() % 89 + 11; // Random number between 11 and 99
// Calculate the quotient
double result = static_cast<double>(y) / x;
// Output the result
std::cout << "Random numbers: x = " << x << ", y = " << y << std::endl;
std::cout << "The value of y/x = " << result << std::endl;
return 0;
}
```
**Conclusion:**
This program demonstrates the process of generating random numbers and performing basic arithmetic operations in C++. Adjusting the random number ranges allows you to experiment and understand how random number generation works in various contexts.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F91500a8e-39cc-457a-a00f-f9674d495f85%2Fda4f3975-9d0a-478e-8419-24689c2f9bb7%2F8umsw0o_processed.jpeg&w=3840&q=75)
Transcribed Image Text:**Title: Generating Random Numbers and Calculating Quotients using C++**
**Objective:**
Learn how to generate random numbers in C++ and perform simple arithmetic operations. This example focuses on generating two random numbers within specified ranges and finding their quotient.
**Task Description:**
Write a C++ program to generate a random integer `x` in the range [1, 9] and another random integer `y` in the range [11, 99]. The program should then calculate and print the value of the quotient `y/x`.
**Implementation Details:**
1. **Import Required Libraries:**
- Use `<iostream>` for input and output.
- Use `<cstdlib>` and `<ctime>` for generating random numbers.
2. **Generate Random Numbers:**
- Initialize the random seed using `srand(time(0))` to ensure different outputs each time the program runs.
- Generate `x` using `rand() % 9 + 1` to ensure `x` is between 1 and 9.
- Generate `y` using `rand() % 89 + 11` for a range between 11 and 99.
3. **Calculate and Print the Quotient:**
- Perform the division of the two random numbers (`y/x`).
- Print the result to the console.
**Sample Code:**
```cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
// Initialize random seed
srand(time(0));
// Generate random numbers
int x = rand() % 9 + 1; // Random number between 1 and 9
int y = rand() % 89 + 11; // Random number between 11 and 99
// Calculate the quotient
double result = static_cast<double>(y) / x;
// Output the result
std::cout << "Random numbers: x = " << x << ", y = " << y << std::endl;
std::cout << "The value of y/x = " << result << std::endl;
return 0;
}
```
**Conclusion:**
This program demonstrates the process of generating random numbers and performing basic arithmetic operations in C++. Adjusting the random number ranges allows you to experiment and understand how random number generation works in various contexts.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
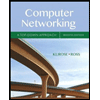
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
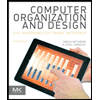
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
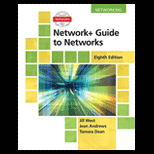
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
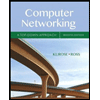
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
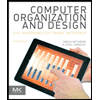
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
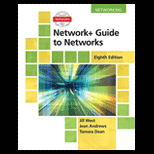
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
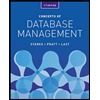
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
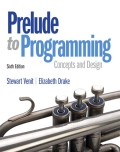
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
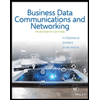
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY