Write a C program Producer – Consumer as a classical problem of synchronization Step 2. Write a program* that solves the producer - consumer problem. You may use the following pseudo code for implementation. *program to write: produce -consumer problem to produce and consume the alphabet. //Shared data: semaphore full, empty, mutex; //pool of n buffers, each can hold one item //mutex provides mutual exclusion to the buffer pool //empty and full count the number of empty and full buffers //Initially: full = 0, empty = n, mutex = 1 //Producer thread do { … produce next item … wait(empty); wait(mutex); … add the item to buffer … signal(mutex); signal(full); } while (1); //Consumer thread do { wait(full) wait(mutex); … remove next item from buffer … signal(mutex); signal(empty); … consume the item } while (1);
Write a C program
Producer – Consumer as a classical problem of synchronization
Step 2. Write a program* that solves the producer - consumer problem. You may use the following pseudo code
for implementation.
*program to write: produce -consumer problem to produce and consume the alphabet.
//Shared data: semaphore full, empty, mutex;
//pool of n buffers, each can hold one item
//mutex provides mutual exclusion to the buffer pool
//empty and full count the number of empty and full buffers
//Initially: full = 0, empty = n, mutex = 1
//Producer thread
do {
…
produce next item
…
wait(empty);
wait(mutex);
…
add the item to buffer
…
signal(mutex);
signal(full);
} while (1);
//Consumer thread
do {
wait(full)
wait(mutex);
…
remove next item from buffer
…
signal(mutex);
signal(empty);
…
consume the item
} while (1);

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

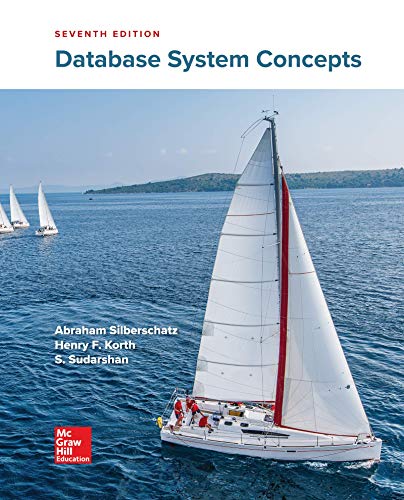
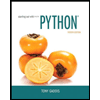
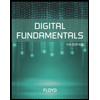
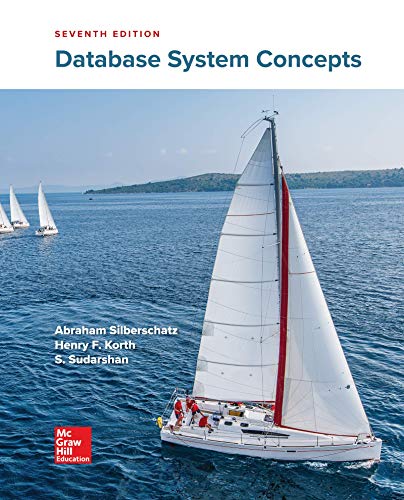
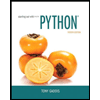
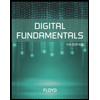
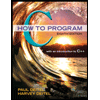
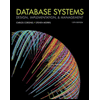
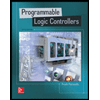