Write a C++ program for encrypting and decrypting files. Since this program performs two different functionalities (encryption and decryption), prompt the user to select the type of cryptographic technique as shown below: Welcome to the Cryptographic Techniques Program Please enter your selection: 1. Encrypt 2. Decrypt When the user selects 1 or 2, s/he will be asked to specify the input and output files. This program will be tested using a .txt file. If the user selects to encrypt the file (i.e. option 1), then the program will encrypt the original text and outputs the encrypted text to a new file. If the user selects to decrypt the file (i.e. option 2), then the program will decrypt the encrypted text and outputs the decrypted text to a new file. The program should give the user the option whether to continue with encrypting/decrypting files (i.e. entering letter �C�) or exit the program (i.e. entering the letter �E�). This program MUST be written using functions and arrays. The program MUST and can ONLY contain the following function definitions and layout: /************************************************************** * This function displays the menu options as in the handout * **************************************************************/ void displayMenu() { /* function body */ } /************************************************************** * This function encrypts the content of infile and saves the * * encrypted text into outfile * * @param infile string (file that has raw text) * * @param outfile string (file that will have encrypted text) * **************************************************************/ void encrypt(string infile, string outfile) { /* function body */ } /************************************************************** * This function decrypts the content of infile and saves the * * decrypted text into outfile * * @param infile string (file that has encrypted text) * * @param outfile string (file that will have decrypted text) * **************************************************************/ void decrypt(string infile, string outfile) { /* function body */ } /************************************************************** * This function takes an character and a cipher key to return * * an encrypted character. * * @param c is a char (the character to be encrypted) * * @param key is an integer (cipher key given in the handout) * **************************************************************/ char ceaserCipher(char c, int key) { /* function body */ } /************************************************************** * This function takes an encrypted character and a cipher key * * to return a decrypted character. * * @param c is a char (the character to be decrypted) * * @param key is an integer (cipher key given in the handout) * **************************************************************/ char ceaserDecipher(char c, int key) { /* function body */ } /************************************************************** * This is the main function of the program. * * @return a value to terminate the program successfully * **************************************************************/ int main() { displayMenu(); // 1. Get user input (e.g. encrypt/decrypt and filenames) // 2. Call the appropriate function(s) to process encryption/decryption // 3. Then, get user input to continue or exit the program return 0; } **Please write clean code. Any code that does not meet the asking requirements will be rated 0 stars.
Write a C++
Welcome to the Cryptographic Techniques Program
Please enter your selection:
1. Encrypt
2. Decrypt
When the user selects 1 or 2, s/he will be asked to specify the input and output files.
This program will be tested using a .txt file.
If the user selects to encrypt the file (i.e. option 1), then the program will encrypt the original text and outputs the encrypted text to a new file.
If the user selects to decrypt the file (i.e. option 2), then the program will decrypt the encrypted text and outputs the decrypted text to a new file.
The program should give the user the option whether to continue with encrypting/decrypting files (i.e. entering letter �C�) or exit the program (i.e. entering the letter �E�).
This program MUST be written using functions and arrays.
The program MUST and can ONLY contain the following function definitions and layout:
/**************************************************************
* This function displays the menu options as in the handout *
**************************************************************/
void displayMenu()
{ /* function body */ }
/**************************************************************
* This function encrypts the content of infile and saves the *
* encrypted text into outfile *
* @param infile string (file that has raw text) *
* @param outfile string (file that will have encrypted text) *
**************************************************************/
void encrypt(string infile, string outfile)
{ /* function body */ }
/**************************************************************
* This function decrypts the content of infile and saves the *
* decrypted text into outfile *
* @param infile string (file that has encrypted text) *
* @param outfile string (file that will have decrypted text) *
**************************************************************/
void decrypt(string infile, string outfile)
{ /* function body */ }
/**************************************************************
* This function takes an character and a cipher key to return *
* an encrypted character. *
* @param c is a char (the character to be encrypted) *
* @param key is an integer (cipher key given in the handout) *
**************************************************************/
char ceaserCipher(char c, int key)
{ /* function body */ }
/**************************************************************
* This function takes an encrypted character and a cipher key *
* to return a decrypted character. *
* @param c is a char (the character to be decrypted) *
* @param key is an integer (cipher key given in the handout) *
**************************************************************/
char ceaserDecipher(char c, int key)
{ /* function body */ }
/**************************************************************
* This is the main function of the program. *
* @return a value to terminate the program successfully *
**************************************************************/
int main()
{
displayMenu();
// 1. Get user input (e.g. encrypt/decrypt and filenames)
// 2. Call the appropriate function(s) to process encryption/decryption
// 3. Then, get user input to continue or exit the program
return 0;
}
**Please write clean code. Any code that does not meet the asking requirements will be rated 0 stars.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 7 images

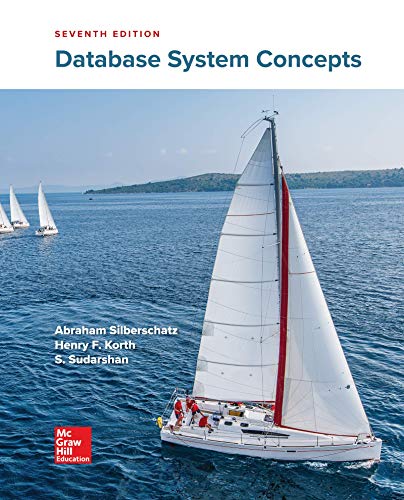
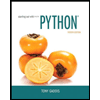
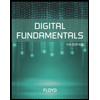
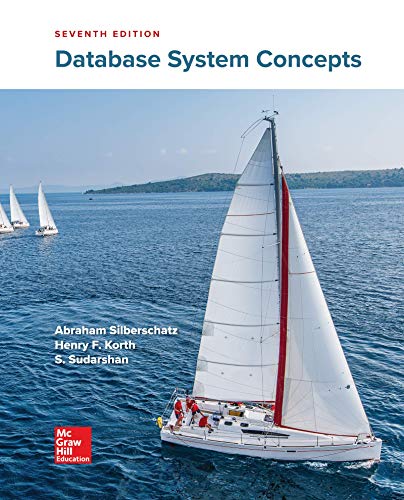
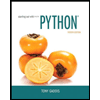
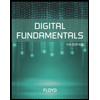
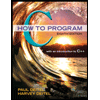
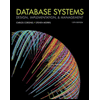
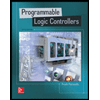