Write a C++ function that checks whether a given string is a palindrome. A palindrome is a word, phrase, or sequence that reads the same forwards and backwards, ignoring spaces, punctuation, and capitalization. Your program should use a combination of a stack and a queue to perform the check. It should return true if the input string is a palindrome and false otherwise. Function Signature bool isPalindrome(const std::string& str); Input A string str (1 <= str.length() <= 10^5) consisting of alphanumeric characters, punctuation and spaces.
Write a C++ function that checks whether a given string is a palindrome.
A palindrome is a word, phrase, or sequence that reads the same forwards and backwards, ignoring spaces, punctuation, and capitalization.
Your program should use a combination of a stack and a queue to perform the check. It should return true if the input string is a palindrome and false otherwise.
Function Signature
bool isPalindrome(const std::string& str);
Input
A string str (1 <= str.length() <= 10^5) consisting of alphanumeric characters, punctuation and spaces.
Output
true if str is a palindrome, false otherwise.
Example
bool result1 = isPalindrome("A man a plan a canal Panama"); // true
bool result2 = isPalindrome("race car"); //
true bool result3 = isPalindrome("hello world"); // false
- Convert the input string to lowercase to ignore capitalization.
- Remove spaces and punctuation from the string to focus only on alphanumeric characters.
- Use a stack to push characters onto it as you iterate through the string.
- Use a queue to enqueue characters as you iterate through the string in reverse.
- Compare characters popped from the stack with characters dequeued from the queue to check if they match.
- Use the C++ Standard Library's std::stack and std::queue for this exercise.
Constraints
Do not use additional data structures or containers beyond the stack and queue for this exercise.
Sample Palindromes (for testing)
- racecar
- deified
- level
- rotor
- 123454321
- Madam in Eden, I'm Adam.
- Was it a car or a cat I saw?

Step by step
Solved in 3 steps with 2 images

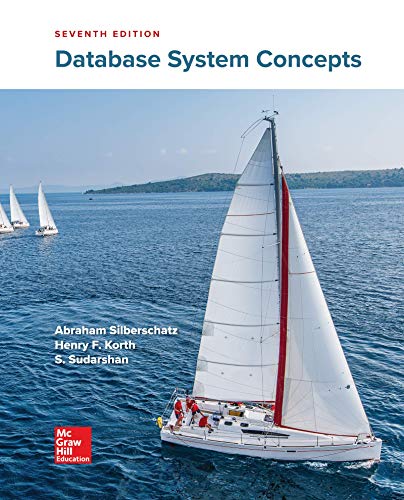
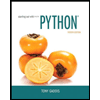
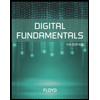
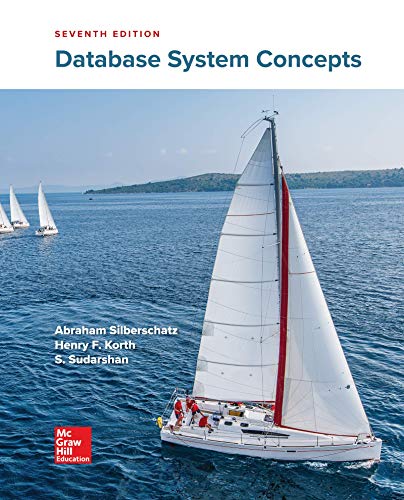
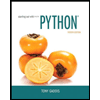
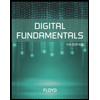
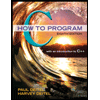
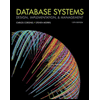
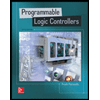