With this coding find the Max Roughness, Root-Mean-Square, and Arithmetic Mean using the values from the Surface.txt: -4.1 -2.2 -0.5 1.2 3.3 4.6 5.1 2.1 0.2 -3.6 -4.1 0.2 0.5 2.2 4.1 -0.2 -1.2 -3.3 -4.6 -5.0 -2.2 -1.1 0.8 3.2 -0.1 -4.8 Coding: import numpy as np import math as mt #declare variables roughness=np.zeros(50,dtype=float) #50 dimension array min=0.0 max=0.0 sum=0.0 sqSum=0.0 Ra=0.0 Rq=0.0 maxRoughness=0.0 i=0 #check if input file exists or not try : #open Surface.txt file in 'read' mode inFile=open("Surface.txt","r") except FileNotFoundError: print("\n\t Error in opening the file... \n\n") else: #read the first value from file roughness[i]=float(inFile.readline()) #initialise min and max values min=max=roughness[i] #initialise sum sum+=roughness[i] #initialise sqSum sqSum+=roughness[i]*roughness[i] #loop till end of file while i<49 : i+=1 #read value from file; one at a time roughness[i]=float(inFile.readline()) #check value is less than min or not if roughness[i]max : max=roughness[i] #calculate cumulative sum sum+=roughness[i] #calculate cumulative sum square if the value sqSum+=roughness[i]*roughness[i] #close inFile inFile.close() #increment i as it started from 0 i+=1 #calculate value of Ra Ra = abs(sum)/i #calculate value of Rq Rq = mt.sqrt(sqSum/i) #calculate value of Maximum roughness maxRoughness = max-min #open Indicators.txt file in append mode outFile=open("Indicators.txt","a") #write message to output file outFile.write("Input data (measures of the roughness of the surface of an object): \n\n") #for formatting outFile.write("\t") #loop to write 6 values per line for x in range(i) : outFile.write(str(roughness[x])+" ") #insert new line after 6 values if (x+1)%6==0 : outFile.write("\n\t") #for formatting outFile.write("\n\n\n") #write the 3 surface roughness indicators to file outFile.write("Arithmetic mean value (Ra) :"+str(Ra)+"\n") outFile.write("Root-mean square average (Rq) :"+str(Rq)+"\n") outFile.write("Maximum roughness height :"+str(maxRoughness)+"\n") outFile.close() #print message print("Calculations completed and the results are stored in Indicators.txt \n")
With this coding find the Max Roughness, Root-Mean-Square, and Arithmetic Mean using the values from the Surface.txt: -4.1 -2.2 -0.5 1.2 3.3 4.6 5.1 2.1 0.2 -3.6 -4.1 0.2 0.5 2.2 4.1 -0.2 -1.2 -3.3 -4.6 -5.0 -2.2 -1.1 0.8 3.2 -0.1 -4.8 Coding: import numpy as np import math as mt #declare variables roughness=np.zeros(50,dtype=float) #50 dimension array min=0.0 max=0.0 sum=0.0 sqSum=0.0 Ra=0.0 Rq=0.0 maxRoughness=0.0 i=0 #check if input file exists or not try : #open Surface.txt file in 'read' mode inFile=open("Surface.txt","r") except FileNotFoundError: print("\n\t Error in opening the file... \n\n") else: #read the first value from file roughness[i]=float(inFile.readline()) #initialise min and max values min=max=roughness[i] #initialise sum sum+=roughness[i] #initialise sqSum sqSum+=roughness[i]*roughness[i] #loop till end of file while i<49 : i+=1 #read value from file; one at a time roughness[i]=float(inFile.readline()) #check value is less than min or not if roughness[i]max : max=roughness[i] #calculate cumulative sum sum+=roughness[i] #calculate cumulative sum square if the value sqSum+=roughness[i]*roughness[i] #close inFile inFile.close() #increment i as it started from 0 i+=1 #calculate value of Ra Ra = abs(sum)/i #calculate value of Rq Rq = mt.sqrt(sqSum/i) #calculate value of Maximum roughness maxRoughness = max-min #open Indicators.txt file in append mode outFile=open("Indicators.txt","a") #write message to output file outFile.write("Input data (measures of the roughness of the surface of an object): \n\n") #for formatting outFile.write("\t") #loop to write 6 values per line for x in range(i) : outFile.write(str(roughness[x])+" ") #insert new line after 6 values if (x+1)%6==0 : outFile.write("\n\t") #for formatting outFile.write("\n\n\n") #write the 3 surface roughness indicators to file outFile.write("Arithmetic mean value (Ra) :"+str(Ra)+"\n") outFile.write("Root-mean square average (Rq) :"+str(Rq)+"\n") outFile.write("Maximum roughness height :"+str(maxRoughness)+"\n") outFile.close() #print message print("Calculations completed and the results are stored in Indicators.txt \n")
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
With this coding find the Max Roughness, Root-Mean-Square, and Arithmetic Mean using the values from the Surface.txt:
-4.1 -2.2 -0.5 1.2 3.3 4.6 5.1 2.1 0.2 -3.6 -4.1 0.2 0.5 2.2 4.1 -0.2 -1.2 -3.3 -4.6 -5.0 -2.2 -1.1 0.8 3.2 -0.1 -4.8
Coding:
import numpy as np
import math as mt
#declare variables
roughness=np.zeros(50,dtype=float) #50 dimension array
min=0.0
max=0.0
sum=0.0
sqSum=0.0
Ra=0.0
Rq=0.0
maxRoughness=0.0
i=0
#check if input file exists or not
try :
#open Surface.txt file in 'read' mode
inFile=open("Surface.txt","r")
except FileNotFoundError:
print("\n\t Error in opening the file... \n\n")
else:
#read the first value from file
roughness[i]=float(inFile.readline())
#initialise min and max values
min=max=roughness[i]
#initialise sum
sum+=roughness[i]
#initialise sqSum
sqSum+=roughness[i]*roughness[i]
#loop till end of file
while i<49 :
i+=1
#read value from file; one at a time
roughness[i]=float(inFile.readline())
#check value is less than min or not
if roughness[i]<min :
min=roughness[i]
#check value is greater than max or not
if roughness[i]>max :
max=roughness[i]
#calculate cumulative sum
sum+=roughness[i]
#calculate cumulative sum square if the value
sqSum+=roughness[i]*roughness[i]
#close inFile
inFile.close()
#increment i as it started from 0
i+=1
#calculate value of Ra
Ra = abs(sum)/i
#calculate value of Rq
Rq = mt.sqrt(sqSum/i)
#calculate value of Maximum roughness
maxRoughness = max-min
#open Indicators.txt file in append mode
outFile=open("Indicators.txt","a")
#write message to output file
outFile.write("Input data (measures of the roughness of the surface of an object): \n\n")
#for formatting
outFile.write("\t")
#loop to write 6 values per line
for x in range(i) :
outFile.write(str(roughness[x])+" ")
#insert new line after 6 values
if (x+1)%6==0 :
outFile.write("\n\t")
#for formatting
outFile.write("\n\n\n")
#write the 3 surface roughness indicators to file
outFile.write("Arithmetic mean value (Ra) :"+str(Ra)+"\n")
outFile.write("Root-mean square average (Rq) :"+str(Rq)+"\n")
outFile.write("Maximum roughness height :"+str(maxRoughness)+"\n")
outFile.close()
#print message
print("Calculations completed and the results are stored in Indicators.txt \n")
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
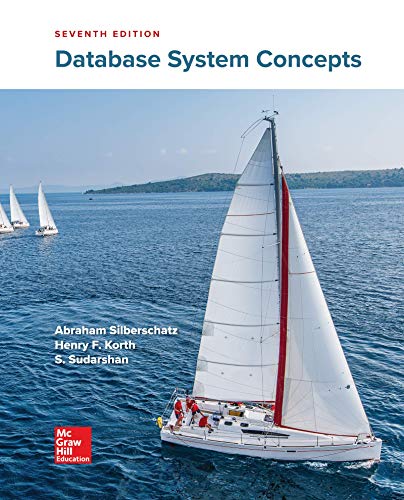
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
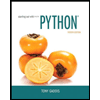
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
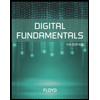
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
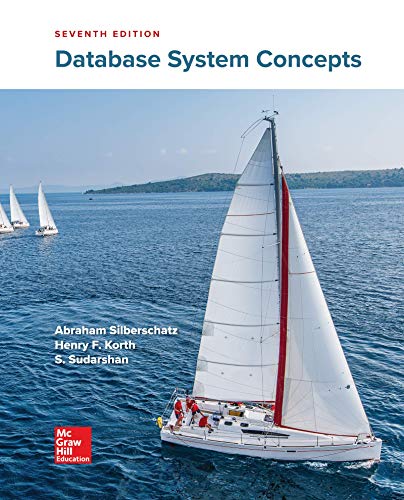
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
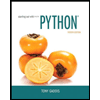
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
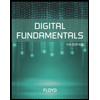
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
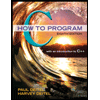
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
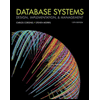
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
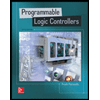
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education