Why wont my code work? Sample code of what the input and out put should be. Hello there! My name is CarBot. What is your name? Jane Hello Jane, it is great to meet you! Jane, where are you from? Washington Washington sounds like a pleasant place to grow up. Hmmmm, what else can I ask you... oh! What year were you born? 1950 You are 67 years old, that makes you 33.5 times as old as I am! I'm only 2 years YOUNG! What is your dream car? BMWi8 Wow, I have always wanted a BMWi8 as well. How much does a BMWi8 cost? 141695 What is a reasonable yearly interest rate (%) on a beautiful car like that? 2.0 And if you had to take out a loan to buy the BMWi8, how many years would you take the loan out for? 5 If you bought the BMWi8, you would have a monthly payment of $2483.60, hopefully that is reasonable for your budget. That's a total of $149015.76 over 5 years! I hope you can make the purchase!
Why wont my code work? Sample code of what the input and out put should be.
Hello there! My name is CarBot. What is your name?
Jane
Hello Jane, it is great to meet you! Jane, where are you from?
Washington
Washington sounds like a pleasant place to grow up. Hmmmm, what else can I ask you... oh! What year were you born?
1950
You are 67 years old, that makes you 33.5 times as old as I am! I'm only 2 years YOUNG! What is your dream car?
BMWi8
Wow, I have always wanted a BMWi8 as well. How much does a BMWi8 cost?
141695
What is a reasonable yearly interest rate (%) on a beautiful car like that?
2.0
And if you had to take out a loan to buy the BMWi8, how many years would you take the loan out for?
5
If you bought the BMWi8, you would have a monthly payment of $2483.60, hopefully that is reasonable for your budget.
That's a total of $149015.76 over 5 years! I hope you can make the purchase!



Below is the C++ code:
1#include <iostream>
2#include <iomanip>
3#include <string>
4#include <cmath>
5
6using namespace std;
7
8int main()
9{
10 // User entry of name
11 string userName;
12 cout << "Hello there! My name is CarBot. What is your name?" << endl;
13 cin >> userName; // Only use first name. You can modify this to allow multi-word
14 cout << "Hello " << userName << " it is great to meet you!" << userName << ", ";
15
16 // Prompt user to input location
17 string userFrom;
18 cout << "where are you from?" << endl;
19 cin >> userFrom; // Only use single word. You can modify this to allow multi-word
20 cout << userFrom << " sounds like a pleasant place to grow up. Hmmmm, what else can I ask you... oh! What year were you born?" << endl;
21
22 // Prompting to enter birth year to calculate age
23 int birthYear = 0;
24 cin >> birthYear;
25 int age = 2023 - birthYear;
26 cout << "You are " << age << " years old, that makes you " << setprecision(2) << fixed << static_cast<double>(age) / 2 << " times as old as I am! I'm only 2 years YOUNG! What is your dream car?" << endl;
27
28 // Dream car string and price of the car for the end calculations
29 string car;
30 cin >> car;
31 cout << "Wow, I have always wanted a " << car << " as well. How much does a " << car << " cost?" << endl;
32
33 double carPrice = 0;
34 cin >> carPrice;
35
36 // Setting up interest rate and for how many years
37 cout << "What is a reasonable yearly interest rate (%) on a beautiful car like that?" << endl;
38 double interest = 0;
39 cin >> interest;
40
41 cout << "And if you had to take out a loan to buy the " << car << ", how many years would you take the loan out for?" << endl;
42 int years = 0;
43 cin >> years;
44
45 int numPayments = -12 * years;
46 double monthlyInterest = interest / 12.0 / 100.0;
47 double monthlyPayment = (carPrice * monthlyInterest) / (1 - pow(1 + monthlyInterest, numPayments));
48 double totalPayment = monthlyPayment * (years * 12);
49
50 // Outputting the monthly and total payment
51 cout << "If you bought the " << car << ", you would have a monthly payment of $" << monthlyPayment << ", hopefully that is reasonable for your budget." << endl;
52 cout << "That's a total of $" << totalPayment << " over " << years << " years!" << " I hope you can make the purchase!" << endl;
53
54 return 0;
55}
56
Step by step
Solved in 3 steps with 1 images

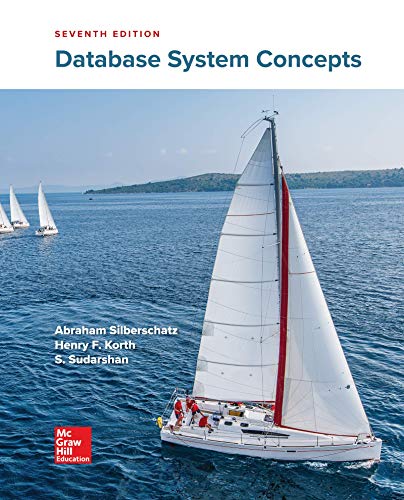
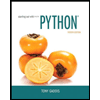
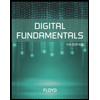
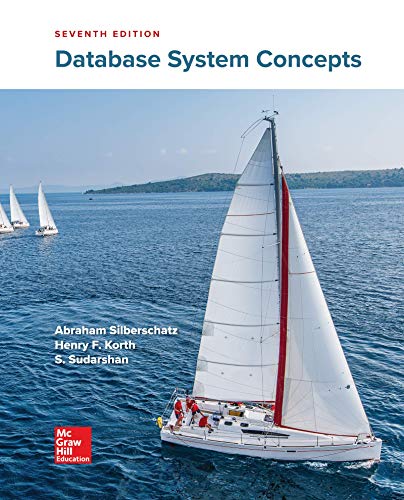
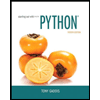
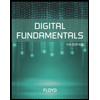
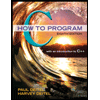
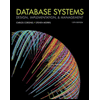
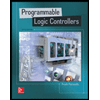