Why when i run my program display this error message 'NoneType' object has no attribute 'find' from bs4 import BeautifulSoup as soup import validators import requests def main(): url = '' while validators.url(url) != True: url = input("Enter valid URL: ") url = 'https://blog.python.org/' filename = url[7:15]+'.txt' try: print("Downloading text from:", url) print("Saving text to:", filename) imported_webpage = getWebPage(url) while True: choice = input("Do you want to view raw Html? (y/n): ") if choice[0].lower() == 'y': print("### Raw HTML ###") print(imported_webpage) print("### End of Raw HTML ###") break elif choice[0].lower() == 'n': break else: print("Invalid Input. Enter valid Input.") poem = extractPoem(imported_webpage) exportFile(poem, filename) print("Page Saved. Re-importing file") poem_content = importFile(filename) print("Displaying File content:") print(poem_content) except Exception as e: print(e) def getWebPage(url): page_html = requests.get(url) page_soup = soup(page_html.text, "html.parser") return page_soup def extractPoem(page_soup): found = page_soup.find("div", {"class": "python"}) # poem = "\n".join(line.strip() for line in found.python.text.split("\n")) poem = "\n".join(line.strip() for line in found.find('python').text.split("\n")) #poem = "\n".join(line.strip() for line in found.find('python').text.split("\n")) return poem def exportFile(content, filename): with open(filename, 'w') as f: f.write(content) def importFile(filename): with open(filename, 'r') as f: content = f.read() return content if __name__ == "__main__": main()
Why when i run my program display this error message
'NoneType' object has no attribute 'find'
from bs4 import BeautifulSoup as soup
import validators
import requests
def main():
url = ''
while validators.url(url) != True:
url = input("Enter valid URL: ")
url = 'https://blog.python.org/'
filename = url[7:15]+'.txt'
try:
print("Downloading text from:", url)
print("Saving text to:", filename)
imported_webpage = getWebPage(url)
while True:
choice = input("Do you want to view raw Html? (y/n): ")
if choice[0].lower() == 'y':
print("### Raw HTML ###")
print(imported_webpage)
print("### End of Raw HTML ###")
break
elif choice[0].lower() == 'n':
break
else:
print("Invalid Input. Enter valid Input.")
poem = extractPoem(imported_webpage)
exportFile(poem, filename)
print("Page Saved. Re-importing file")
poem_content = importFile(filename)
print("Displaying File content:")
print(poem_content)
except Exception as e:
print(e)
def getWebPage(url):
page_html = requests.get(url)
page_soup = soup(page_html.text, "html.parser")
return page_soup
def extractPoem(page_soup):
found = page_soup.find("div", {"class": "python"})
# poem = "\n".join(line.strip() for line in found.python.text.split("\n"))
poem = "\n".join(line.strip() for line in found.find('python').text.split("\n"))
#poem = "\n".join(line.strip() for line in found.find('python').text.split("\n"))
return poem
def exportFile(content, filename):
with open(filename, 'w') as f:
f.write(content)
def importFile(filename):
with open(filename, 'r') as f:
content = f.read()
return content
if __name__ == "__main__":
main()

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

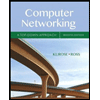
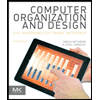
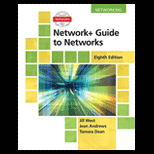
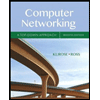
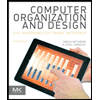
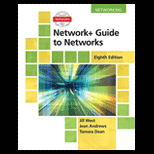
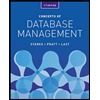
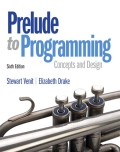
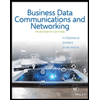